Tizen Native API
|
ResourceImage is an image loaded using a URL. More...
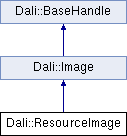
Public Types | |
typedef Signal< void(ResourceImage) > | ResourceImageSignal |
Type of signal for LoadingFinished and Uploaded. | |
Public Member Functions | |
ResourceImage () | |
Constructor which creates an empty ResourceImage object. | |
~ResourceImage () | |
Destructor. | |
ResourceImage (const ResourceImage &handle) | |
This copy constructor is required for (smart) pointer semantics. | |
ResourceImage & | operator= (const ResourceImage &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
LoadingState | GetLoadingState () const |
Query whether the image data has loaded. | |
std::string | GetUrl () const |
Returns the URL of the image. | |
void | Reload () |
Reload image from filesystem. | |
ResourceImageSignal & | LoadingFinishedSignal () |
Emitted when the image data loads successfully, or when the loading fails. | |
Static Public Member Functions | |
static ImageDimensions | GetImageSize (const std::string &url) |
Get the size of an image from disk. | |
static ResourceImage | DownCast (BaseHandle handle) |
Downcast a handle to ResourceImage handle. | |
ResourceImageFactoryFunctions | |
Create ResourceImage object instances using these functions. | |
static ResourceImage | New (const std::string &url, bool orientationCorrection=true) |
Create an initialised ResourceImage object. | |
static ResourceImage | New (const std::string &url, ImageDimensions size, FittingMode::Type fittingMode=FittingMode::DEFAULT, SamplingMode::Type samplingMode=SamplingMode::DEFAULT, bool orientationCorrection=true) |
Create an initialised ResourceImage object. |
Detailed Description
ResourceImage is an image loaded using a URL.
ResourceImage Loading
When the ResourceImage is created, resource loading will be attempted unless the ResourceImage is created with ResourceImage::IMMEDIATE loading policy or a compatible resource is found in cache. In case of loading images ResourceImage::ON_DEMAND, resource loading will only be attempted if the associated Dali::Toolkit::ImageView is put on Stage. Scaling of images to a desired smaller size can be requested by providing desired dimensions, scaling mode and filter mode to to ResourceImage::New().
Custom load requests
Size, scaling mode, filter mode, and orientation compensation can be set when requesting an image.
Compatible resources
Before loading a new ResourceImage the internal image resource cache is checked by dali. If there is an image already loaded in memory and is deemed "compatible" with the requested image, that resource is reused. This happens for example if a loaded image exists with the same URL, scaling and filtering modes, and the difference between both of the dimensions is less than a few pixels.
Reloading images
The same request used on creating the ResourceImage is re-issued when reloading images. If the file changed since the last load operation, this might result in a different resource. Reload only takes effect if both of these conditions apply:
- The ResourceImage has already finished loading
- The ResourceImage is either on Stage or using ResourceImage::IMMEDIATE load policy
- Since :
- 2.4
Member Typedef Documentation
typedef Signal< void (ResourceImage) > Dali::ResourceImage::ResourceImageSignal |
Type of signal for LoadingFinished and Uploaded.
- Since :
- 2.4
Constructor & Destructor Documentation
Constructor which creates an empty ResourceImage object.
Use ResourceImage::New(...) to create an initialised object.
- Since :
- 2.4
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since :
- 2.4
Dali::ResourceImage::ResourceImage | ( | const ResourceImage & | handle | ) |
This copy constructor is required for (smart) pointer semantics.
- Since :
- 2.4
- Parameters:
-
[in] handle A reference to the copied handle
Member Function Documentation
static ResourceImage Dali::ResourceImage::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcast a handle to ResourceImage handle.
If handle points to a ResourceImage object the downcast produces valid handle. If not the returned handle is left uninitialized.
- Since :
- 2.4
- Parameters:
-
[in] handle Handle to an object
Reimplemented from Dali::Image.
static ImageDimensions Dali::ResourceImage::GetImageSize | ( | const std::string & | url | ) | [static] |
Get the size of an image from disk.
This function will read the header info from file on disk and is synchronous, so it should not be used repeatedly or in tight loops.
- Since :
- 2.4
- Parameters:
-
[in] url The URL of the image file.
- Returns:
- The width and height in pixels of the image.
std::string Dali::ResourceImage::GetUrl | ( | ) | const |
Returns the URL of the image.
- Since :
- 2.4
- Returns:
- The URL of the image file.
Emitted when the image data loads successfully, or when the loading fails.
- Since :
- 2.4
- Returns:
- A signal object to Connect() with.
static ResourceImage Dali::ResourceImage::New | ( | const std::string & | url, |
bool | orientationCorrection = true |
||
) | [static] |
Create an initialised ResourceImage object.
Uses defaults for all options.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/internet
- Remarks:
- http://tizen.org/privilege/internet is needed if url is a http or https address.
- Parameters:
-
[in] url The URL of the image file to use (can be a local file path, http or https address). [in] orientationCorrection Reorient the image to respect any orientation metadata in its header.
- Returns:
- A handle to a newly allocated object
static ResourceImage Dali::ResourceImage::New | ( | const std::string & | url, |
ImageDimensions | size, | ||
FittingMode::Type | fittingMode = FittingMode::DEFAULT , |
||
SamplingMode::Type | samplingMode = SamplingMode::DEFAULT , |
||
bool | orientationCorrection = true |
||
) | [static] |
Create an initialised ResourceImage object.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/internet
- Remarks:
- http://tizen.org/privilege/internet is needed if url is a http or https address.
- Parameters:
-
[in] url The URL of the image file to use (can be a local file path, http or https address). [in] size The width and height to fit the loaded image to. [in] fittingMode The method used to fit the shape of the image before loading to the shape defined by the size parameter. [in] samplingMode The filtering method used when sampling pixels from the input image while fitting it to desired size. [in] orientationCorrection Reorient the image to respect any orientation metadata in its header.
- Returns:
- A handle to a newly allocated object
ResourceImage& Dali::ResourceImage::operator= | ( | const ResourceImage & | rhs | ) |
This assignment operator is required for (smart) pointer semantics.
- Since :
- 2.4
- Parameters:
-
[in] rhs A reference to the copied handle
- Returns:
- A reference to this
void Dali::ResourceImage::Reload | ( | ) |
Reload image from filesystem.
The original set of image loading attributes (requested dimensions, scaling mode and filter mode) are used when requesting the image again.
- Since :
- 2.4
- Note:
- If image is offstage and OnDemand policy is set, the reload request is ignored.