Tizen Native API
|
Functions | |
int | player_create (player_h *player) |
Creates a player handle for playing multimedia content. | |
int | player_destroy (player_h player) |
Destroys the media player handle and releases all its resources. | |
int | player_prepare (player_h player) |
Prepares the media player for playback. | |
int | player_prepare_async (player_h player, player_prepared_cb callback, void *user_data) |
Prepares the media player for playback, asynchronously. | |
int | player_unprepare (player_h player) |
Resets the media player. | |
int | player_set_uri (player_h player, const char *uri) |
Sets the data source (file-path, HTTP or RSTP URI) to use. | |
int | player_set_display (player_h player, player_display_type_e type, player_display_h display) |
Sets the video display. | |
int | player_start (player_h player) |
Starts or resumes playback. | |
int | player_stop (player_h player) |
Stops playing media content. | |
int | player_pause (player_h player) |
Pauses the player. | |
int | player_set_memory_buffer (player_h player, const void *data, int size) |
Sets memory as the data source. | |
int | player_get_state (player_h player, player_state_e *state) |
Gets the player's current state. | |
int | player_set_volume (player_h player, float left, float right) |
Sets the player's volume. | |
int | player_get_volume (player_h player, float *left, float *right) |
Gets the player's current volume factor. | |
int | player_set_sound_type (player_h player, sound_type_e type) |
Sets the player's volume type. | |
int | player_set_audio_latency_mode (player_h player, audio_latency_mode_e latency_mode) |
Sets the audio latency mode. | |
int | player_get_audio_latency_mode (player_h player, audio_latency_mode_e *latency_mode) |
Gets the current audio latency mode. | |
int | player_set_play_position (player_h player, int millisecond, bool accurate, player_seek_completed_cb callback, void *user_data) |
Sets the seek position for playback, asynchronously. | |
int | player_get_play_position (player_h player, int *millisecond) |
Gets the current position in milliseconds. | |
int | player_set_mute (player_h player, bool muted) |
Sets the player's mute status. | |
int | player_is_muted (player_h player, bool *muted) |
Gets the player's mute status. | |
int | player_set_looping (player_h player, bool looping) |
Sets the player's looping status. | |
int | player_is_looping (player_h player, bool *looping) |
Gets the player's looping status. | |
int | player_set_completed_cb (player_h player, player_completed_cb callback, void *user_data) |
Registers a callback function to be invoked when the playback is finished. | |
int | player_unset_completed_cb (player_h player) |
Unregisters the callback function. | |
int | player_set_interrupted_cb (player_h player, player_interrupted_cb callback, void *user_data) |
Registers a callback function to be invoked when the playback is interrupted or the interrupt is completed. | |
int | player_unset_interrupted_cb (player_h player) |
Unregisters the callback function. | |
int | player_set_error_cb (player_h player, player_error_cb callback, void *user_data) |
Registers a callback function to be invoked when an error occurs. | |
int | player_unset_error_cb (player_h player) |
Unregisters the callback function. | |
int | player_capture_video (player_h player, player_video_captured_cb callback, void *user_data) |
Captures the video frame, asynchronously. | |
int | player_set_media_packet_video_frame_decoded_cb (player_h player, player_media_packet_video_decoded_cb callback, void *user_data) |
Registers a media packet video callback function to be called once per frame. | |
int | player_unset_media_packet_video_frame_decoded_cb (player_h player) |
Unregisters the callback function. | |
int | player_set_streaming_cookie (player_h player, const char *cookie, int size) |
Sets the cookie for streaming playback. | |
int | player_set_streaming_user_agent (player_h player, const char *user_agent, int size) |
Sets the streaming user agent for playback. | |
int | player_get_streaming_download_progress (player_h player, int *start, int *current) |
Gets the download progress for streaming playback. | |
int | player_set_buffering_cb (player_h player, player_buffering_cb callback, void *user_data) |
Registers a callback function to be invoked when there is a change in the buffering status of a media stream. | |
int | player_unset_buffering_cb (player_h player) |
Unregisters the callback function. | |
int | player_set_progressive_download_path (player_h player, const char *path) |
Sets a path to download, progressively. | |
int | player_get_progressive_download_status (player_h player, unsigned long *current, unsigned long *total_size) |
Gets the status of progressive download. | |
int | player_set_progressive_download_message_cb (player_h player, player_pd_message_cb callback, void *user_data) |
Registers a callback function to be invoked when progressive download is started or completed. | |
int | player_unset_progressive_download_message_cb (player_h player) |
Unregisters the callback function. | |
int | player_set_playback_rate (player_h player, float rate) |
Sets the playback rate. | |
int | player_set_video_stream_changed_cb (player_h player, player_video_stream_changed_cb callback, void *user_data) |
Registers a callback function to be invoked when video stream is changed. | |
int | player_unset_video_stream_changed_cb (player_h player) |
Unregisters the video stream changed callback function. | |
int | player_get_track_count (player_h player, player_stream_type_e type, int *count) |
Gets the track count. | |
int | player_select_track (player_h player, player_stream_type_e type, int index) |
Selects a track to play. | |
int | player_get_current_track (player_h player, player_stream_type_e type, int *index) |
Gets current track index. | |
int | player_get_track_language_code (player_h player, player_stream_type_e type, int index, char **code) |
Gets language code of a track. | |
int | player_push_media_stream (player_h player, media_packet_h packet) |
Pushes elementary stream to decode audio or video. | |
int | player_set_media_stream_info (player_h player, player_stream_type_e type, media_format_h format) |
Sets contents information for media stream. | |
int | player_set_media_stream_buffer_status_cb (player_h player, player_stream_type_e type, player_media_stream_buffer_status_cb callback, void *user_data) |
Registers a callback function to be invoked when buffer underrun or overflow is occurred. | |
int | player_unset_media_stream_buffer_status_cb (player_h player, player_stream_type_e type) |
Unregisters the buffer status callback function. | |
int | player_set_media_stream_seek_cb (player_h player, player_stream_type_e type, player_media_stream_seek_cb callback, void *user_data) |
Registers a callback function to be invoked when seeking is occurred. | |
int | player_unset_media_stream_seek_cb (player_h player, player_stream_type_e type) |
Unregisters the seek callback function. | |
int | player_set_media_stream_buffer_max_size (player_h player, player_stream_type_e type, unsigned long long max_size) |
Sets the max size bytes of buffer. | |
int | player_get_media_stream_buffer_max_size (player_h player, player_stream_type_e type, unsigned long long *max_size) |
Gets the max size bytes of buffer. | |
int | player_set_media_stream_buffer_min_threshold (player_h player, player_stream_type_e type, unsigned int percent) |
Sets the buffer threshold percent of buffer. | |
int | player_get_media_stream_buffer_min_threshold (player_h player, player_stream_type_e type, unsigned int *percent) |
Gets the buffer threshold percent of buffer. | |
Typedefs | |
typedef struct player_s * | player_h |
The media player's type handle. | |
typedef void * | player_display_h |
The player display handle. | |
typedef void(* | player_prepared_cb )(void *user_data) |
Called when the media player is prepared. | |
typedef void(* | player_completed_cb )(void *user_data) |
Called when the media player is completed. | |
typedef void(* | player_seek_completed_cb )(void *user_data) |
Called when the seek operation is completed. | |
typedef void(* | player_interrupted_cb )(player_interrupted_code_e code, void *user_data) |
Called when the media player is interrupted. | |
typedef void(* | player_error_cb )(int error_code, void *user_data) |
Called when an error occurs in the media player. | |
typedef void(* | player_buffering_cb )(int percent, void *user_data) |
Called when the buffering percentage of the media playback is updated. | |
typedef void(* | player_pd_message_cb )(player_pd_message_type_e type, void *user_data) |
Called when progressive download is started or completed. | |
typedef void(* | player_video_captured_cb )(unsigned char *data, int width, int height, unsigned int size, void *user_data) |
Called when the video is captured. | |
typedef void(* | player_media_packet_video_decoded_cb )(media_packet_h pkt, void *user_data) |
Called to register for notifications about delivering media packet when every video frame is decoded. | |
typedef void(* | player_media_stream_buffer_status_cb )(player_media_stream_buffer_status_e status, void *user_data) |
Called when the buffer level drops below the threshold of max size or no free space in buffer. | |
typedef void(* | player_media_stream_seek_cb )(unsigned long long offset, void *user_data) |
Called to notify the next push-buffer offset when seeking is occurred. | |
typedef void(* | player_video_stream_changed_cb )(int width, int height, int fps, int bit_rate, void *user_data) |
Called to notify the video stream changed. | |
Defines | |
#define | GET_DISPLAY(x) (void*)(x) |
Definition for a display handle from evas object. |
The Player API provides functions for media playback and controlling media playback attributes.
Required Header
#include <player.h>
Overview
The Player API provides a way to play multimedia content. Content can be played from a file, from the network, or from memory. It gives the ability to start/stop/pause/mute, set the playback position (that is, seek), perform various status queries, and control the display.
Additional functions allow registering notifications via callback functions for various state change events.
This API also enables collaboration with the GUI service to present a video.
State Diagram
Playback of multimedia content is controlled by a state machine. The following diagram shows the life cycle and states of the Player.
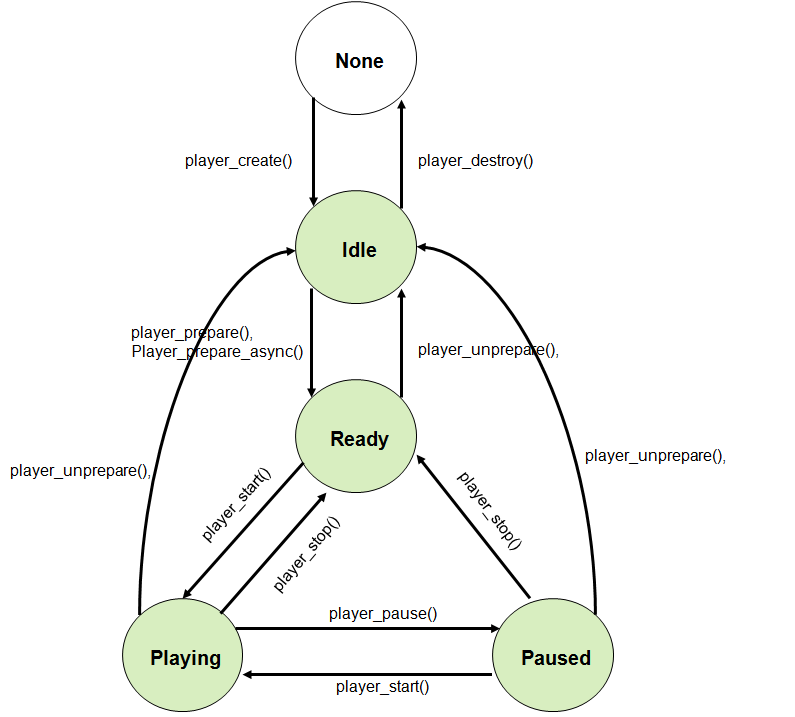
State Transitions
FUNCTION | PRE-STATE | POST-STATE | SYNC TYPE |
---|---|---|---|
player_create() | NONE | IDLE | SYNC |
player_destroy() | IDLE | NONE | SYNC |
player_prepare() | IDLE | READY | SYNC |
player_prepare_async() | IDLE | READY | ASYNC |
player_unprepare() | READY, PLAYING or PAUSED | IDLE | SYNC |
player_start() | READY or PAUSED | PLAYING | SYNC |
player_stop() | PLAYING | READY | SYNC |
player_pause() | PLAYING | PAUSED | SYNC |
State Dependent Function Calls
The following table shows state-dependent function calls. It is forbidden to call the functions listed below in wrong states. Violation of this rule may result in unpredictable behavior.
FUNCTION | VALID STATES | DESCRIPTION |
---|---|---|
player_create() | ANY | - |
player_destroy() | ANY | - |
player_prepare() | IDLE | This function must be called after player_create() |
player_unprepare() | READY/PAUSED/PLAYING | This function must be called after player_stop() or player_start() or player_pause() |
player_start() | READY/ PAUSED | This function must be called after player_prepare() |
player_stop() | PLAYING/ PAUSED | This function must be called after player_start() or player_pause() |
player_pause() | PLAYING | This function must be called after player_start() |
player_set_completed_cb() player_set_interrupted_cb() player_set_error_cb() player_set_buffering_cb() player_set_subtitle_updated_cb() | IDLE/ READY/ PLAYING/ PAUSED | This function must be called after player_create() |
player_unset_completed_cb() player_unset_interrupted_cb() player_unset_error_cb() player_unset_buffering_cb() player_unset_subtitle_updated_cb() | IDLE/ READY/ PLAYING/ PAUSED | This function must be called after register callback functions such as player_set_completed_cb() |
player_get_state() | ANY | - |
player_set_uri() | IDLE | This function must be called before player_prepare() |
player_set_memory_buffer() | IDLE | This function must be called before player_prepare() |
player_set_subtitle_path() | IDLE | This function must be called before player_prepare() |
player_set_volume() | IDLE/ READY/ PLAYING/ PAUSED | This function must be called after player_create() |
player_get_volume() | IDLE/ READY/ PLAYING/ PAUSED | This function must be called after player_create() |
player_set_sound_type() | IDLE | This function must be called after player_create() |
player_set_mute() | IDLE/ READY/ PLAYING/ PAUSED | This function must be called after player_create() |
player_is_muted() | IDLE/ READY/ PLAYING/ PAUSED | This function must be called after player_create() |
player_set_looping() | IDLE/ READY/ PLAYING/ PAUSED | This function must be called after player_create() |
player_is_looping() | IDLE/ READY/ PLAYING/ PAUSED | This function must be called after player_create() |
player_get_duration() | PLAYING/ PAUSED | This function must be called after player_start() |
player_set_display() | IDLE | This function must be called before player_prepare() |
player_set_display_mode() player_set_display_visible() | IDLE/ READY/ PLAYING/ PAUSED | This function must be called after player_create() |
player_get_display_rotation() player_is_display_visible() | IDLE/ READY/ PLAYING/ PAUSED | This function must be called after player_create() |
player_get_video_size() | READY/ PLAYING/ PAUSED | This function must be called after player_prepare() |
Asynchronous Operations
All functions that change the player state are synchronous except player_prepare_async(), player_set_play_position(), and player_capture_video(). Thus the result is passed to the application via the callback mechanism.
Callback(Event) Operations
REGISTER | UNREGISTER | CALLBACK | DESCRIPTION |
---|---|---|---|
player_set_completed_cb() | player_unset_completed_cb() | player_completed_cb() | called when playback is completed |
player_set_interrupted_cb() | player_unset_interrupted_cb() | player_interrupted_cb() | called when playback is interrupted by player_interrupted_code_e |
player_set_error_cb() | player_unset_error_cb() | player_error_cb() | called when an error has occurred |
player_set_buffering_cb() | player_unset_buffering_cb() | player_buffering_cb() | called during content buffering |
player_set_progressive_download_message_cb() | player_unset_progressive_download_message_cb() | player_pd_message_cb() | called when a progressive download starts or completes |
player_set_subtitle_updated_cb() | player_unset_subtitle_updated_cb() | player_subtitle_updated_cb() | called when a subtitle updates |
player_set_media_packet_video_frame_decoded_cb() | player_unset_media_packet_video_frame_decoded_cb() | player_media_packet_video_decoded_cb() | called when a video frame is decoded |
Define Documentation
#define GET_DISPLAY | ( | x | ) | (void*)(x) |
Definition for a display handle from evas object.
- Since :
- 2.3
Typedef Documentation
typedef void(* player_buffering_cb)(int percent, void *user_data) |
Called when the buffering percentage of the media playback is updated.
- Since :
- 2.3
If the buffer is full, it will return 100%.
- Parameters:
-
[in] percent The percentage of buffering completed (0~100) [in] user_data The user data passed from the callback registration function
typedef void(* player_completed_cb)(void *user_data) |
Called when the media player is completed.
- Since :
- 2.3
It will be invoked when player has reached the end of the stream.
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- Precondition:
- It will be invoked when the playback is completed if you register this callback using player_set_completed_cb().
typedef void* player_display_h |
The player display handle.
- Since :
- 2.3
typedef void(* player_error_cb)(int error_code, void *user_data) |
Called when an error occurs in the media player.
- Since :
- 2.3
- Parameters:
-
[in] error_code The error code [in] user_data The user data passed from the callback registration function
typedef struct player_s* player_h |
The media player's type handle.
- Since :
- 2.3
typedef void(* player_interrupted_cb)(player_interrupted_code_e code, void *user_data) |
Called when the media player is interrupted.
- Since :
- 2.3
- Parameters:
-
[in] error_code The interrupted error code [in] user_data The user data passed from the callback registration function
typedef void(* player_media_packet_video_decoded_cb)(media_packet_h pkt, void *user_data) |
Called to register for notifications about delivering media packet when every video frame is decoded.
- Since :
- 2.3
- Remarks:
- This function is issued in the context of gstreamer so the UI update code should not be directly invoked.
the packet should be released by media_packet_destroy() after use.
Otherwises, decoder can't work more because it can't have enough buffer to fill decoded frame.
- Parameters:
-
[in] pkt Reference pointer to the media packet [in] user_data The user data passed from the callback registration function
typedef void(* player_media_stream_buffer_status_cb)(player_media_stream_buffer_status_e status, void *user_data) |
Called when the buffer level drops below the threshold of max size or no free space in buffer.
- Since :
- 2.4
- Remarks:
- This API is used for media stream playback only.
- Parameters:
-
[in] user_data The user data passed from the callback registration function
typedef void(* player_media_stream_seek_cb)(unsigned long long offset, void *user_data) |
Called to notify the next push-buffer offset when seeking is occurred.
- Since :
- 2.4
- Remarks:
- This API is used for media stream playback only.
The next push-buffer should produce buffers from the new offset.
- Parameters:
-
[in] offset The new byte position to seek [in] user_data The user data passed from the callback registration function
typedef void(* player_pd_message_cb)(player_pd_message_type_e type, void *user_data) |
Called when progressive download is started or completed.
- Since :
- 2.3
- Parameters:
-
[in] type The message type for progressive download [in] user_data The user data passed from the callback registration function
typedef void(* player_prepared_cb)(void *user_data) |
Called when the media player is prepared.
- Since :
- 2.3
It will be invoked when player has reached the begin of stream.
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- Precondition:
- player_prepare_async() will cause this callback.
- Postcondition:
- The player state will be PLAYER_STATE_READY.
- See also:
- player_prepare_async()
typedef void(* player_seek_completed_cb)(void *user_data) |
Called when the seek operation is completed.
- Since :
- 2.3
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- See also:
- player_set_play_position()
typedef void(* player_video_captured_cb)(unsigned char *data, int width, int height, unsigned int size, void *user_data) |
Called when the video is captured.
- Since :
- 2.3
- Remarks:
- The color space format of the captured image is IMAGE_UTIL_COLORSPACE_RGB888.
- Parameters:
-
[in] data The captured image buffer [in] width The width of the captured image [in] height The height of the captured image [in] size The size of the captured image [in] user_data The user data passed from the callback registration function
- See also:
- player_capture_video()
typedef void(* player_video_stream_changed_cb)(int width, int height, int fps, int bit_rate, void *user_data) |
Called to notify the video stream changed.
- Since :
- 2.4
The video stream changing is detected just before rendering operation.
- Parameters:
-
[in] width The width of the captured image [in] height The height of the captured image [in] fps The frame per second of the video
It can be0
if there is no video stream information.[in] bit_rate The video bit rate [Hz]
It can be an invalid value if there is no video stream information.[in] user_data The user data passed from the callback registration function
- See also:
- player_set_video_stream_changed_cb()
Enumeration Type Documentation
enum audio_latency_mode_e |
enum player_error_e |
Enumeration for media player's error codes.
- Since :
- 2.3
- Enumerator:
Enumeration for media player's interruption type.
- Since :
- 2.3
- Enumerator:
Enumeration of media stream buffer status.
- Since :
- 2.4
enum player_state_e |
Enumeration for media player state.
- Since :
- 2.3
enum player_stream_type_e |
Function Documentation
int player_capture_video | ( | player_h | player, |
player_video_captured_cb | callback, | ||
void * | user_data | ||
) |
Captures the video frame, asynchronously.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation Video type should be set using player_set_display() otherwises, audio stream is only processed even though video file is set. PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_PLAYING by calling player_start() or set to PLAYER_STATE_PAUSED by calling player_pause().
- Postcondition:
- It invokes player_video_captured_cb() when capture completes, if you set a callback.
- See also:
- player_video_captured_cb()
int player_create | ( | player_h * | player | ) |
Creates a player handle for playing multimedia content.
- Since :
- 2.3
- Remarks:
- You must release player by using player_destroy().
Although you can create multiple player handles at the same time, the player cannot guarantee proper operation because of limited resources, such as audio or display device.
- Parameters:
-
[out] player A new handle to the media player
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_OUT_OF_MEMORY Out of memory PLAYER_ERROR_INVALID_OPERATION Invalid operation
- Postcondition:
- The player state will be PLAYER_STATE_IDLE.
- See also:
- player_destroy()
int player_destroy | ( | player_h | player | ) |
Destroys the media player handle and releases all its resources.
- Since :
- 2.3
- Remarks:
- To completely shutdown player operation, call this function with a valid player handle from any player state.
- Parameters:
-
[in] player The handle to the media player to be destroyed
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE.
- Postcondition:
- The player state will be PLAYER_STATE_NONE.
- See also:
- player_create()
int player_get_audio_latency_mode | ( | player_h | player, |
audio_latency_mode_e * | latency_mode | ||
) |
Gets the current audio latency mode.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [out] latency_mode The latency mode to get from the audio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
int player_get_current_track | ( | player_h | player, |
player_stream_type_e | type, | ||
int * | index | ||
) |
Gets current track index.
- Since :
- 2.4
Index starts from 0.
- Remarks:
- PLAYER_STREAM_TYPE_VIDEO is not supported.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [out] index The index of track
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state #PLAYER_ERROR_NOT_SUPPORTD Not supported
- Precondition:
- The player state must be one of these: PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED
int player_get_media_stream_buffer_max_size | ( | player_h | player, |
player_stream_type_e | type, | ||
unsigned long long * | max_size | ||
) |
Gets the max size bytes of buffer.
- Since :
- 2.4
- Remarks:
- This API is used for media stream playback only.
- If the buffer level over the max size, player_media_stream_buffer_status_cb() will be invoked with overflow status.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [out] max_size The max bytes of buffer
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
int player_get_media_stream_buffer_min_threshold | ( | player_h | player, |
player_stream_type_e | type, | ||
unsigned int * | percent | ||
) |
Gets the buffer threshold percent of buffer.
- Since :
- 2.4
- Remarks:
- This API is used for media stream playback only.
- If the buffer level drops below the percent value, player_media_stream_buffer_status_cb() will be invoked with underrun status.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [out] percent The minimum threshold(0~100) of buffer
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
int player_get_play_position | ( | player_h | player, |
int * | millisecond | ||
) |
Gets the current position in milliseconds.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [out] millisecond The current position in milliseconds
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_SEEK_FAILED Seek operation failure
- Precondition:
- The player state must be one of these: PLAYER_STATE_IDLE, PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- See also:
- player_set_play_position()
int player_get_progressive_download_status | ( | player_h | player, |
unsigned long * | current, | ||
unsigned long * | total_size | ||
) |
Gets the status of progressive download.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [out] current The current download position (bytes) [out] total_size The total size of the file (bytes)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The progressive download path must be set by calling player_set_progressive_download_path().
- The player state must be set to PLAYER_STATE_PLAYING by calling player_start() or set to PLAYER_STATE_PAUSED by calling player_pause().
int player_get_state | ( | player_h | player, |
player_state_e * | state | ||
) |
Gets the player's current state.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [out] state The current state of the player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- See also:
- player_state_e
int player_get_streaming_download_progress | ( | player_h | player, |
int * | start, | ||
int * | current | ||
) |
Gets the download progress for streaming playback.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [out] start The starting position in percentage [0, 100] [out] current The current position in percentage [0, 100]
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_PLAYING by calling player_start() or set to PLAYER_STATE_PAUSED by calling player_pause().
int player_get_track_count | ( | player_h | player, |
player_stream_type_e | type, | ||
int * | count | ||
) |
Gets the track count.
- Since :
- 2.4
- Remarks:
- PLAYER_STREAM_TYPE_VIDEO is not supported.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [out] count The number of track
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state #PLAYER_ERROR_NOT_SUPPORTD Not supported
- Precondition:
- The player state must be one of these: PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED
int player_get_track_language_code | ( | player_h | player, |
player_stream_type_e | type, | ||
int | index, | ||
char ** | code | ||
) |
Gets language code of a track.
- Since :
- 2.4
- Remarks:
- code must be released with
free()
by caller - PLAYER_STREAM_TYPE_VIDEO is not supported.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [in] index The index of track [out] code A language code in ISO 639-1. "und" will be returned if the language is undefined.
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state #PLAYER_ERROR_NOT_SUPPORTD Not supported
- Precondition:
- The player state must be one of these: PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED
int player_get_volume | ( | player_h | player, |
float * | left, | ||
float * | right | ||
) |
Gets the player's current volume factor.
- Since :
- 2.3
The range of left and right is from 0
to 1.0
, inclusive (1.0 = 100%). This function gets the player volume, not the system volume. To get the system volume, use the Sound Manager API.
- Parameters:
-
[in] player The handle to the media player [out] left The current left volume scalar [out] right The current right volume scalar
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_volume()
int player_is_looping | ( | player_h | player, |
bool * | looping | ||
) |
Gets the player's looping status.
- Since :
- 2.3
If the looping status is true
, playback automatically restarts upon finishing. If it is false
, it won't.
- Parameters:
-
[in] player The handle to the media player [out] looping The looping status: ( true
= looping,false
= non-looping )
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be one of these: PLAYER_STATE_IDLE, PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- See also:
- player_set_looping()
int player_is_muted | ( | player_h | player, |
bool * | muted | ||
) |
Gets the player's mute status.
- Since :
- 2.3
If the mute status is true
, no sounds are played. If it is false
, sounds are played at the previously set volume level.
- Parameters:
-
[in] player The handle to the media player [out] muted The current mute status: ( true
= mute,false
= not muted)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- Precondition:
- The player state must be one of these: PLAYER_STATE_IDLE, PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- See also:
- player_set_mute()
int player_pause | ( | player_h | player | ) |
Pauses the player.
- Since :
- 2.3
- Remarks:
- You can resume playback using player_start().
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid state PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_SOUND_POLICY Sound policy error
- Precondition:
- The player state must be set to PLAYER_STATE_PLAYING.
- Postcondition:
- The player state will be PLAYER_STATE_READY.
- See also:
- player_start()
int player_prepare | ( | player_h | player | ) |
Prepares the media player for playback.
- Since :
- 2.3
- Remarks:
- The mediastorage privilege(http://tizen.org/privilege/mediastorage) should be added if any video/audio files are used to play located in the internal storage.
- The externalstorage privilege(http://tizen.org/privilege/externalstorage) should be added if any video/audio files are used to play located in the external storage.
- The internet privilege(http://tizen.org/privilege/internet) should be added if any URLs are used to play from network.
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_URI Invalid URI PLAYER_ERROR_NO_SUCH_FILE File not found PLAYER_ERROR_NOT_SUPPORTED_FILE File not supported PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare(). After that, call player_set_uri() to load the media content you want to play.
- Postcondition:
- The player state will be PLAYER_STATE_READY.
int player_prepare_async | ( | player_h | player, |
player_prepared_cb | callback, | ||
void * | user_data | ||
) |
Prepares the media player for playback, asynchronously.
- Since :
- 2.3
- Remarks:
- The mediastorage privilege(http://tizen.org/privilege/mediastorage) should be added if any video/audio files are used to play located in the internal storage.
- The externalstorage privilege(http://tizen.org/privilege/externalstorage) should be added if any video/audio files are used to play located in the external storage.
- The internet privilege(http://tizen.org/privilege/internet) should be added if any URLs are used to play from network.
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_URI Invalid URI PLAYER_ERROR_NO_SUCH_FILE File not found PLAYER_ERROR_NOT_SUPPORTED_FILE File not supported PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare(). After that, call player_set_uri() to load the media content you want to play.
- Postcondition:
- It invokes player_prepared_cb() when playback is prepared.
int player_push_media_stream | ( | player_h | player, |
media_packet_h | packet | ||
) |
Pushes elementary stream to decode audio or video.
- Since :
- 2.4
- Remarks:
- player_set_media_stream_info() should be called before using this API.
- Parameters:
-
[in] player The handle to media player [in] packet The media packet to decode
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid state PLAYER_ERROR_NOT_SUPPORTED_FILE File not supported
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE at least.
- See also:
- player_set_media_stream_info()
int player_select_track | ( | player_h | player, |
player_stream_type_e | type, | ||
int | index | ||
) |
Selects a track to play.
- Since :
- 2.4
- Remarks:
- PLAYER_STREAM_TYPE_VIDEO is not supported.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [in] index The index of track
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state #PLAYER_ERROR_NOT_SUPPORTD Not supported
- Precondition:
- The player state must be one of these: PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED
- See also:
- player_get_current_track()
int player_set_audio_latency_mode | ( | player_h | player, |
audio_latency_mode_e | latency_mode | ||
) |
Sets the audio latency mode.
- Since :
- 2.3
- Remarks:
- The default audio latency mode of the player is AUDIO_LATENCY_MODE_MID. To get the current audio latency mode, use player_get_audio_latency_mode(). If it's high mode, audio output interval can be increased so, it can keep more audio data to play. But, state transition like pause or resume can be more slower than default(mid) mode.
- Parameters:
-
[in] player The handle to the media player [in] latency_mode The latency mode to be applied to the audio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
int player_set_buffering_cb | ( | player_h | player, |
player_buffering_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be invoked when there is a change in the buffering status of a media stream.
- Since :
- 2.3
- Remarks:
- The media resource should be streamed over the network.
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_FEATURE_NOT_SUPPORTED_ON_DEVICE Unsupported feature
- Postcondition:
- player_buffering_cb() will be invoked.
int player_set_completed_cb | ( | player_h | player, |
player_completed_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be invoked when the playback is finished.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- Postcondition:
- player_completed_cb() will be invoked.
int player_set_display | ( | player_h | player, |
player_display_type_e | type, | ||
player_display_h | display | ||
) |
Sets the video display.
- Since :
- 2.3
- Remarks:
- To get display to set, use GET_DISPLAY().
- To use the multiple surface display mode, use player_set_display() again with a different display type.
-
We are not supporting changing display between different types.
If you want to change display handle after calling player_prepare(), you must use the same display type as what you set before.
- Parameters:
-
[in] player The handle to the media player [in] type The display type [in] display The handle to display
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be one of these: PLAYER_STATE_IDLE, PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- See also:
- player_set_display_rotation
int player_set_error_cb | ( | player_h | player, |
player_error_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be invoked when an error occurs.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- Postcondition:
- player_error_cb() will be invoked.
- See also:
- player_unset_error_cb()
- player_error_cb()
int player_set_interrupted_cb | ( | player_h | player, |
player_interrupted_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be invoked when the playback is interrupted or the interrupt is completed.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- Postcondition:
- player_interrupted_cb() will be invoked.
int player_set_looping | ( | player_h | player, |
bool | looping | ||
) |
Sets the player's looping status.
- Since :
- 2.3
If the looping status is true
, playback automatically restarts upon finishing. If it is false
, it won't. The default value is false
.
- Parameters:
-
[in] player The handle to the media player [in] looping The new looping status: ( true
= looping,false
= non-looping )
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be one of these: PLAYER_STATE_IDLE, PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- See also:
- player_is_looping()
int player_set_media_packet_video_frame_decoded_cb | ( | player_h | player, |
player_media_packet_video_decoded_cb | callback, | ||
void * | user_data | ||
) |
Registers a media packet video callback function to be called once per frame.
- Since :
- 2.3
- Remarks:
- This function should be called before preparing.
A registered callback is called on the internal thread of the player.
A video frame can be retrieved using a registered callback as a media packet.
The callback function holds the same buffer that will be drawn on the display device.
So if you change the media packet in a registerd callback, it will be displayed on the device
and the media packet is available until it's destroyed by media_packet_destroy().
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to be registered [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid state
- Precondition:
- The player's state should be PLAYER_STATE_IDLE. And, PLAYER_DISPLAY_TYPE_NONE should be set by calling player_set_display.
int player_set_media_stream_buffer_max_size | ( | player_h | player, |
player_stream_type_e | type, | ||
unsigned long long | max_size | ||
) |
Sets the max size bytes of buffer.
- Since :
- 2.4
- Remarks:
- This API is used for media stream playback only.
- If the buffer level over the max size, player_media_stream_buffer_status_cb() will be invoked with overflow status.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [in] max_size The max bytes of buffer
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
int player_set_media_stream_buffer_min_threshold | ( | player_h | player, |
player_stream_type_e | type, | ||
unsigned int | percent | ||
) |
Sets the buffer threshold percent of buffer.
- Since :
- 2.4
- Remarks:
- This API is used for media stream playback only.
- If the buffer level drops below the percent value, player_media_stream_buffer_status_cb() will be invoked with underrun status.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [in] percent The minimum threshold(0~100) of buffer
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
int player_set_media_stream_buffer_status_cb | ( | player_h | player, |
player_stream_type_e | type, | ||
player_media_stream_buffer_status_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be invoked when buffer underrun or overflow is occurred.
- Since :
- 2.4
- Remarks:
- This API is used for media stream playback only.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [in] callback The buffer status callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- Postcondition:
- player_media_stream_buffer_status_cb() will be invoked.
int player_set_media_stream_info | ( | player_h | player, |
player_stream_type_e | type, | ||
media_format_h | format | ||
) |
Sets contents information for media stream.
- Since :
- 2.4
- Remarks:
- AV format should be set before pushing elementary stream with player_push_media_stream().
- AAC can be supported.
- H.264 can be supported.
-
This API should be called before calling the player_prepare() or player_prepare_async()
to reflect the media information when pipeline is created.
- Parameters:
-
[in] player The handle to media player [in] type The type of target stream [in] format The media format to set audio information
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid state
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- See also:
- player_push_media_stream()
int player_set_media_stream_seek_cb | ( | player_h | player, |
player_stream_type_e | type, | ||
player_media_stream_seek_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be invoked when seeking is occurred.
- Since :
- 2.4
- Remarks:
- This API is used for media stream playback only.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- Postcondition:
- player_media_stream_seek_cb() will be invoked.
int player_set_memory_buffer | ( | player_h | player, |
const void * | data, | ||
int | size | ||
) |
Sets memory as the data source.
Associates media content, cached in memory, with the player. Unlike the case of player_set_uri(), the media resides in memory. If the function call is successful, subsequent calls to player_prepare() and player_start() will start playing the media.
- Since :
- 2.3
- Remarks:
- If you provide an invalid data, you won't receive an error message until you call player_start().
- Parameters:
-
[in] player The handle to the media player [in] data The memory pointer of media data [in] size The size of media data
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- See also:
- player_set_uri()
int player_set_mute | ( | player_h | player, |
bool | muted | ||
) |
Sets the player's mute status.
- Since :
- 2.3
If the mute status is true
, no sounds are played. If it is false
, sounds are played at the previously set volume level. Until this function is called, by default the player is not muted.
- Parameters:
-
[in] player The handle to the media player [in] muted The new mute status: ( true
= mute,false
= not muted)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- Precondition:
- The player state must be one of these: PLAYER_STATE_IDLE, PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- See also:
- player_is_muted()
int player_set_play_position | ( | player_h | player, |
int | millisecond, | ||
bool | accurate, | ||
player_seek_completed_cb | callback, | ||
void * | user_data | ||
) |
Sets the seek position for playback, asynchronously.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] millisecond The position in milliseconds from the start to the seek point [in] accurate If true
the selected position is returned, but this might be considerably slow, otherwisefalse
[in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_SEEK_FAILED Seek operation failure
- Precondition:
- The player state must be one of these: PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- Postcondition:
- It invokes player_seek_completed_cb() when seek operation completes, if you set a callback.
- See also:
- player_get_play_position()
int player_set_playback_rate | ( | player_h | player, |
float | rate | ||
) |
Sets the playback rate.
- Since :
- 2.3
The default value is 1.0
.
- Remarks:
- PLAYER_ERROR_INVALID_OPERATION occurs when streaming playback.
-
No operation is performed, if rate is
0
. -
The sound is muted, when playback rate is under
0.0
and over2.0
.
- Parameters:
-
[in] player The handle to the media player [in] rate The playback rate (-5.0x ~ 5.0x)
- Return values:
-
PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_PLAYING by calling player_start().
- The player state must be set to PLAYER_STATE_READY by calling player_prepare() or set to PLAYER_STATE_PLAYING by calling player_start() or set to PLAYER_STATE_PAUSED by calling player_pause().
int player_set_progressive_download_message_cb | ( | player_h | player, |
player_pd_message_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be invoked when progressive download is started or completed.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_FEATURE_NOT_SUPPORTED_ON_DEVICE Unsupported feature
- Precondition:
- The path to download must be set by calling player_set_progressive_download_path().
- Postcondition:
- player_pd_message_cb() will be invoked.
int player_set_progressive_download_path | ( | player_h | player, |
const char * | path | ||
) |
Sets a path to download, progressively.
- Since :
- 2.3
- Remarks:
- Progressive download will be started when you invoke player_start().
- Parameters:
-
[in] player The handle to the media player [in] path The absolute path to download
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_FEATURE_NOT_SUPPORTED_ON_DEVICE Unsupported feature
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
int player_set_sound_type | ( | player_h | player, |
sound_type_e | type | ||
) |
Sets the player's volume type.
- Since :
- 2.3
- Remarks:
- The default sound type of the player is SOUND_TYPE_MEDIA. To get the current sound type, use sound_manager_get_current_sound_type().
- Parameters:
-
[in] player The handle to the media player [in] type The sound type
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create().
int player_set_streaming_cookie | ( | player_h | player, |
const char * | cookie, | ||
int | size | ||
) |
Sets the cookie for streaming playback.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] cookie The cookie to set [in] size The size of the cookie
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- See also:
- player_set_streaming_user_agent()
int player_set_streaming_user_agent | ( | player_h | player, |
const char * | user_agent, | ||
int | size | ||
) |
Sets the streaming user agent for playback.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] user_agent The user agent to set [in] size The size of the user agent
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- See also:
- player_set_streaming_cookie()
int player_set_uri | ( | player_h | player, |
const char * | uri | ||
) |
Sets the data source (file-path, HTTP or RSTP URI) to use.
Associates media contents, referred to by the URI, with the player. If the function call is successful, subsequent calls to player_prepare() and player_start() will start playing the media.
- Since :
- 2.3
- Remarks:
- If you use HTTP or RSTP, URI should start with "http://" or "rtsp://". The default protocol is "file://". If you provide an invalid URI, you won't receive an error message until you call player_start().
- Parameters:
-
[in] player The handle to the media player [in] uri The content location, such as the file path, the URI of the HTTP or RSTP stream you want to play
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- See also:
- player_set_memory_buffer()
int player_set_video_stream_changed_cb | ( | player_h | player, |
player_video_stream_changed_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be invoked when video stream is changed.
- Since :
- 2.4
- Remarks:
- The stream changing is detected just before rendering operation.
- Parameters:
-
[in] player The handle to the media player [in] callback The stream changed callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- Postcondition:
- player_video_stream_changed_cb() will be invoked.
int player_set_volume | ( | player_h | player, |
float | left, | ||
float | right | ||
) |
Sets the player's volume.
- Since :
- 2.3
Setting this volume adjusts the player's instance volume, not the system volume. The valid range is from 0 to 1.0, inclusive (1.0 = 100%). Default value is 1.0. To change system volume, use the Sound Manager API. Finally, it does not support to set other value into each channel currently.
- Parameters:
-
[in] player The handle to the media player [in] left The left volume scalar [in] right The right volume scalar
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_get_volume()
int player_start | ( | player_h | player | ) |
Starts or resumes playback.
- Since :
- 2.3
Plays current media content, or resumes play if paused.
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_CONNECTION_FAILED Network connection failed PLAYER_ERROR_SOUND_POLICY Sound policy error
- Precondition:
- player_prepare() must be called before calling this function.
- The player state must be set to PLAYER_STATE_READY by calling player_prepare() or set to PLAYER_STATE_PAUSED by calling player_pause().
- Postcondition:
- The player state will be PLAYER_STATE_PLAYING.
- It invokes player_completed_cb() when playback completes, if you set a callback with player_set_completed_cb().
- It invokes player_pd_message_cb() when progressive download starts or completes, if you set a download path with player_set_progressive_download_path() and a callback with player_set_progressive_download_message_cb().
int player_stop | ( | player_h | player | ) |
Stops playing media content.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid state PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_SOUND_POLICY Sound policy error
- Precondition:
- The player state must be set to PLAYER_STATE_PLAYING by calling player_start() or set to PLAYER_STATE_PAUSED by calling player_pause().
- Postcondition:
- The player state will be PLAYER_STATE_READY.
- The downloading will be aborted if you use progressive download.
- See also:
- player_start()
- player_pause()
int player_unprepare | ( | player_h | player | ) |
Resets the media player.
The most recently used media is reset and no longer associated with the player. Playback is no longer possible. If you want to use the player again, you will have to set the data URI and call player_prepare() again.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state should be higher than PLAYER_STATE_IDLE.
- Postcondition:
- The player state will be PLAYER_STATE_IDLE.
- See also:
- player_prepare()
int player_unset_buffering_cb | ( | player_h | player | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_buffering_cb()
int player_unset_completed_cb | ( | player_h | player | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_completed_cb()
int player_unset_error_cb | ( | player_h | player | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_error_cb()
int player_unset_interrupted_cb | ( | player_h | player | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_interrupted_cb()
int player_unset_media_packet_video_frame_decoded_cb | ( | player_h | player | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- Precondition:
- The player's state should be PLAYER_STATE_READY or PLAYER_STATE_IDLE
int player_unset_media_stream_buffer_status_cb | ( | player_h | player, |
player_stream_type_e | type | ||
) |
Unregisters the buffer status callback function.
- Since :
- 2.4
- Remarks:
- This API is used for media stream playback only.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
int player_unset_media_stream_seek_cb | ( | player_h | player, |
player_stream_type_e | type | ||
) |
Unregisters the seek callback function.
- Since :
- 2.4
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- See also:
- player_set_media_stream_seek_cb()
int player_unset_progressive_download_message_cb | ( | player_h | player | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
int player_unset_video_stream_changed_cb | ( | player_h | player | ) |
Unregisters the video stream changed callback function.
- Since :
- 2.4
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- See also:
- player_set_video_stream_changed_cb()