Tizen Native API
|
Functions | |
Eina_Array * | eina_array_new (unsigned int step) |
Create a new array. | |
void | eina_array_free (Eina_Array *array) |
Free an array. | |
void | eina_array_step_set (Eina_Array *array, unsigned int sizeof_eina_array, unsigned int step) |
Set the step of an array. | |
static void | eina_array_clean (Eina_Array *array) |
Clean an array. | |
void | eina_array_flush (Eina_Array *array) |
Flush an array. | |
Eina_Bool | eina_array_remove (Eina_Array *array, Eina_Bool(*keep)(void *data, void *gdata), void *gdata) 2) |
Rebuild an array by specifying the data to keep. | |
static Eina_Bool | eina_array_push (Eina_Array *array, const void *data) 2) |
Append a data to an array. | |
static void * | eina_array_pop (Eina_Array *array) |
Remove the last data of an array. | |
static void * | eina_array_data_get (const Eina_Array *array, unsigned int idx) |
Return the data at a given position in an array. | |
static void | eina_array_data_set (const Eina_Array *array, unsigned int idx, const void *data) |
Set the data at a given position in an array. | |
static unsigned int | eina_array_count_get (const Eina_Array *array) |
Return the number of elements in an array. | |
static unsigned int | eina_array_count (const Eina_Array *array) |
Return the number of elements in an array. | |
Eina_Iterator * | eina_array_iterator_new (const Eina_Array *array) |
Get a new iterator associated to an array. | |
Eina_Accessor * | eina_array_accessor_new (const Eina_Array *array) |
Get a new accessor associated to an array. | |
static Eina_Bool | eina_array_foreach (Eina_Array *array, Eina_Each_Cb cb, void *fdata) |
Provide a safe way to iterate over an array. | |
Typedefs | |
typedef struct _Eina_Array | Eina_Array |
typedef void ** | Eina_Array_Iterator |
Defines | |
#define | EINA_ARRAY_ITER_NEXT(array, index, item, iterator) |
Macro to iterate over an array easily. |
These functions provide array management.
The Array data type in Eina is designed to have very fast access to its data (compared to the Eina List). On the other hand, data can be added or removed only at the end of the array. To insert data at any position, the Eina List is the correct container to use.
To use the array data type, Eina must be initialized before any other array functions. When no more eina array functions are used, Eina must be shut down to free all the resources.
An array must be created with eina_array_new(). It allocates all the necessary data for an array. When not needed anymore, an array is freed with eina_array_free(). This frees the memory used by the Eina_Array itself, but does not free any memory used to store the data of each element. To free that memory you must iterate over the array and free each data element individually. A convenient way to do that is by using EINA_ARRAY_ITER_NEXT. An example of that pattern is given in the description of EINA_ARRAY_ITER_NEXT.
- Warning:
- Functions do not check if the used array is valid or not. It's up to the user to be sure of that. It is designed like that for performance reasons.
The usual features of an array are classic ones: to append an element, use eina_array_push() and to remove the last element, use eina_array_pop(). To retrieve the element at a given position, use eina_array_data_get(). The number of elements can be retrieved with eina_array_count().
Eina_Array is different from a conventional C array in a number of ways, most importantly they grow and shrink dynamically, this means that if you add an element to a full array it grows and that when you remove an element from an array it may shrink.
Allocating memory is expensive, so when the array needs to grow it allocates enough memory to hold step
additonal elements, not just the element currently being added. Similarly if you remove elements, it won't free space until you have removed step
elements.
The following image illustrates how an Eina_Array grows:
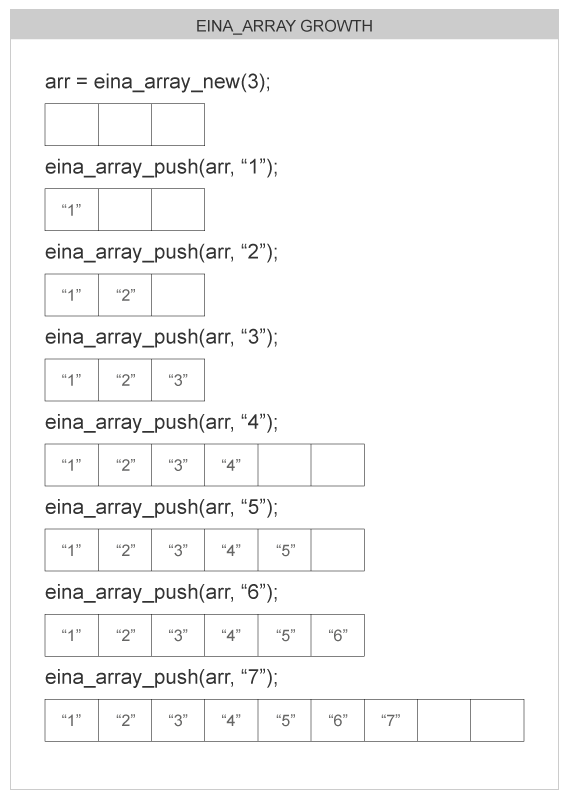
Eina_Array only stores pointers but it can store data of any type in the form of void pointers.
See here some examples:
Define Documentation
#define EINA_ARRAY_ITER_NEXT | ( | array, | |
index, | |||
item, | |||
iterator | |||
) |
for (index = 0, iterator = (array)->data; \ (index < eina_array_count(array)) && ((item = *((iterator)++))); \ ++(index))
Macro to iterate over an array easily.
- Parameters:
-
array The array to iterate over. index The integer number that is increased while iterating. item The data iterator The iterator
This macro allows the iteration over array
in an easy way. It iterates from the first element to the last one. index
is an integer that increases from 0 to the number of elements. item
is the data of each element of array
, so it is a pointer to a type chosen by the user. iterator
is of type Eina_Array_Iterator.
This macro can be used for freeing the data of an array, like in the following example:
Eina_Array *array; char *item; Eina_Array_Iterator iterator; unsigned int i; // array is already filled, // its elements are just duplicated strings, // EINA_ARRAY_ITER_NEXT will be used to free those strings EINA_ARRAY_ITER_NEXT(array, i, item, iterator) free(item);
Typedef Documentation
Type for a generic vector.
Type for an iterator on arrays, used with EINA_ARRAY_ITER_NEXT.
Function Documentation
Eina_Accessor* eina_array_accessor_new | ( | const Eina_Array * | array | ) |
Get a new accessor associated to an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array.
- Returns:
- A new accessor.
- Remarks:
- This function returns a newly allocated accessor associated to
array
. Ifarray
isNULL
or the count member ofarray
is less or equal than 0, this function returnsNULL
. If the memory can not be allocated,NULL
is returned. Otherwise, a valid accessor is returned.
static void eina_array_clean | ( | Eina_Array * | array | ) | [static] |
Clean an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array to clean.
- Remarks:
- This function sets the count member of
array
to 0, however it doesn't free any space. This is particularly useful if you need to empty the array and add lots of elements quickly. For performance reasons, there is no check ofarray
. If it isNULL
or invalid, the program may crash.
static unsigned int eina_array_count | ( | const Eina_Array * | array | ) | [static] |
Return the number of elements in an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array.
- Returns:
- The number of elements.
- Remarks:
- This function returns the number of elements in
array
(array->count). For performance reasons, there is no check ofarray
. If it isNULL
or invalid, the program may crash.
static unsigned int eina_array_count_get | ( | const Eina_Array * | array | ) | [static] |
Return the number of elements in an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array.
- Returns:
- The number of elements.
- Remarks:
- This function returns the number of elements in
array
(array->count). For performance reasons, there is no check ofarray
. If it isNULL
or invalid, the program may crash.
- Deprecated:
- use eina_array_count() Deprecated since Tizen 2.4
static void* eina_array_data_get | ( | const Eina_Array * | array, |
unsigned int | idx | ||
) | [static] |
Return the data at a given position in an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array. [in] idx The potition of the data to retrieve.
- Returns:
- The retrieved data.
- Remarks:
- This function returns the data at the position
idx
inarray
. For performance reasons, there is no check ofarray
oridx
. If it isNULL
or invalid, the program may crash.
static void eina_array_data_set | ( | const Eina_Array * | array, |
unsigned int | idx, | ||
const void * | data | ||
) | [static] |
Set the data at a given position in an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array. [in] idx The position of the data to set. [in] data The data to set.
- Remarks:
- This function sets the data at the position
idx
inarray
todata
, this effectively replaces the previously held data, you must therefore get a pointer to it first if you need to free it. For performance reasons, there is no check ofarray
oridx
. If it isNULL
or invalid, the program may crash.
void eina_array_flush | ( | Eina_Array * | array | ) |
Flush an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array to flush.
- Remarks:
- This function sets the count and total members of
array
to 0, frees and set to NULL its data member. For performance reasons, there is no check ofarray
. If it isNULL
or invalid, the program may crash.
static Eina_Bool eina_array_foreach | ( | Eina_Array * | array, |
Eina_Each_Cb | cb, | ||
void * | fdata | ||
) | [static] |
Provide a safe way to iterate over an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array to iterate over. [in] cb The callback to call for each item. [in] fdata The user data to pass to the callback.
- Returns:
- EINA_TRUE if it successfully iterate all items of the array.
- Remarks:
- This function provides a safe way to iterate over an array.
cb
should return EINA_TRUE as long as you want the function to continue iterating. Ifcb
returns EINA_FALSE, iterations will stop and the function will also return EINA_FALSE.
void eina_array_free | ( | Eina_Array * | array | ) |
Free an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array to free.
- Remarks:
- This function frees
array
. It calls first eina_array_flush() then free the memory of the pointer. It does not free the memory allocated for the elements ofarray
. To free them, walk the array with EINA_ARRAY_ITER_NEXT. For performance reasons, there is no check ofarray
.
Eina_Iterator* eina_array_iterator_new | ( | const Eina_Array * | array | ) |
Get a new iterator associated to an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array.
- Returns:
- A new iterator.
- Remarks:
- This function returns a newly allocated iterator associated to
array
. Ifarray
isNULL
or the count member ofarray
is less or equal than 0, this function returnsNULL
. If the memory can not be allocated,NULL
is returned. Otherwise, a valid iterator is returned.
Eina_Array* eina_array_new | ( | unsigned int | step | ) |
Create a new array.
- Since :
- 2.3
- Parameters:
-
[in] step The count of pointers to add when increasing the array size.
- Returns:
NULL
on failure, nonNULL
otherwise.
- Remarks:
- This function creates a new array. When adding an element, the array allocates
step
elements. When that buffer is full, then adding another element will increase the buffer bystep
elements again. -
This function return a valid array on success, or
NULL
if memory allocation fails.
static void* eina_array_pop | ( | Eina_Array * | array | ) | [static] |
Remove the last data of an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array.
- Returns:
- The retrieved data.
- Remarks:
- This function removes the last data of
array
, decreases the count ofarray
and returns the data. For performance reasons, there is no check ofarray
. If it isNULL
or invalid, the program may crash. If the count member is less or equal than 0,NULL
is returned.
static Eina_Bool eina_array_push | ( | Eina_Array * | array, |
const void * | data | ||
) | [static] |
Append a data to an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array. [in] data The data to add.
- Returns:
- EINA_TRUE on success, EINA_FALSE otherwise.
- Remarks:
- This function appends
data
toarray
. For performance reasons, there is no check ofarray
. If it isNULL
or invalid, the program may crash. Ifdata
isNULL
, or if an allocation is necessary and fails, EINA_FALSE is returned Otherwise, EINA_TRUE is returned.
Eina_Bool eina_array_remove | ( | Eina_Array * | array, |
Eina_Bool(*)(void *data, void *gdata) | keep, | ||
void * | gdata | ||
) |
Rebuild an array by specifying the data to keep.
- Since :
- 2.3
- Parameters:
-
[in] array The array. [in] keep The functions which selects the data to keep. [in] gdata The data to pass to the function keep.
- Returns:
- EINA_TRUE on success, EINA_FALSE otherwise.
- Remarks:
- This function rebuilds
array
be specifying the elements to keep with the functionkeep
. No empty/invalid fields are left in the array.gdata
is an additional data to pass tokeep
. For performance reasons, there is no check ofarray
. If it isNULL
or invalid, the program may crash. - If it wasn't able to remove items due to an allocation failure, it will return EINA_FALSE.
void eina_array_step_set | ( | Eina_Array * | array, |
unsigned int | sizeof_eina_array, | ||
unsigned int | step | ||
) |
Set the step of an array.
- Since :
- 2.3
- Parameters:
-
[in] array The array. [in] sizeof_eina_array Should be the value returned by sizeof(Eina_Array). [in] step The count of pointers to add when increasing the array size.
- Remarks:
- This function sets the step of
array
tostep
. For performance reasons, there is no check ofarray
. If it isNULL
or invalid, the program may crash.
- Warning:
- This function can only be called on uninitialized arrays.