Tizen Native API
6.0
|
The StreamRecorder API provides functions for StreamRecorder with buffer including media packet.
Required Header
#include <streamrecorder.h>
Overview
The StreamRecorder API allows application developers to support using the video or audio recorder. It includes functions that record video or audio and supports to set up notifications for state changes of creation, prepared, record, pause, information about resolution and binary data format, and functions for artistic.
The StreamRecorder API allows creation of components required in recording video or audio including:
- selecting a proper output format
- controlling the StreamRecorder state
- getting supported formats and video resolutions
The StreamRecorder API also notifies you (by callback mechanism) when a significant parameter changes.
Difference
Basically, the StreamRecorder API can help easily to make video/audio content same as the Recorder API.
However, the StreamRecorder API is different from Recorder API.
The StreamRecorder API is able to record user buffer from application. This allows the user to make unique contents with a purpose.
Difference | Recorder | StreamRecorder |
---|---|---|
INPUT | Camera / Mic Device | Media Packet / Buffer |
State Diagram
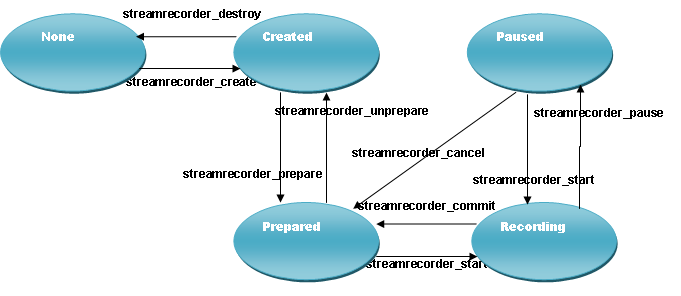
State Transitions
FUNCTION | PRE-STATE | POST-STATE | SYNC TYPE |
---|---|---|---|
streamrecorder_create() | NONE | CREATED | SYNC |
streamrecorder_prepare() | CREATED | PREPARED | SYNC |
streamrecorder_start() | PREPARED / PAUSED | RECORDING | SYNC |
streamrecorder_pause() | RECORDING | PAUSED | SYNC |
streamrecorder_commit() | RECORDING/ PAUSED | PREPARED | SYNC |
streamrecorder_cancel() | RECORDING/ PAUSED | PREPARED | SYNC |
streamrecorder_unprepare() | PREPARED | CREATED | SYNC |
streamrecorder_destroy() | CREATED | NONE | SYNC |
Callback(Event) Operations
The callback mechanism is used to notify the application about significant StreamRecorder events.
REGISTER | UNREGISTER | CALLBACK | DESCRIPTION |
---|---|---|---|
streamrecorder_set_error_cb() | streamrecorder_unset_error_cb() | streamrecorder_error_cb() | This callback is used to notify error has occurred |
Foreach Operations
FOREACH | CALLBACK | DESCRIPTION |
---|---|---|
streamrecorder_foreach_supported_audio_encoder() | streamrecorder_supported_audio_encoder_cb() | Supported audio encoders |
streamrecorder_foreach_supported_video_encoder() | streamrecorder_supported_video_encoder_cb() | Supported video encoders |
streamrecorder_foreach_supported_file_format() | streamrecorder_supported_file_format_cb() | Supported file formats |
streamrecorder_foreach_supported_video_resolution() | streamrecorder_supported_video_resolution_cb() | Supported video resolutions |
Related Features
This API is related with the following feature
It is recommended to design feature related codes in your application for reliability. You can check if a device supports the related features for this API by using System Information, thereby controlling the procedure of your application. To ensure your application is only running on the device with specific features, please define the features in your manifest file using the manifest editor in the SDK. For more information about featuring your application, see Feature Element.
Functions | |
int | streamrecorder_create (streamrecorder_h *recorder) |
Creates a streamrecorder handle to record a video or audio. | |
int | streamrecorder_destroy (streamrecorder_h recorder) |
Destroys the streamrecorder handle. | |
int | streamrecorder_prepare (streamrecorder_h recorder) |
Prepares the streamrecorder for recording. | |
int | streamrecorder_unprepare (streamrecorder_h recorder) |
Resets the streamrecorder. | |
int | streamrecorder_start (streamrecorder_h recorder) |
Starts the recording. | |
int | streamrecorder_pause (streamrecorder_h recorder) |
Pauses the recording. | |
int | streamrecorder_commit (streamrecorder_h recorder) |
Stops recording and saves the result. | |
int | streamrecorder_cancel (streamrecorder_h recorder) |
Cancels the recording. | |
int | streamrecorder_push_stream_buffer (streamrecorder_h recorder, media_packet_h inbuf) |
Pushes buffer to StreamRecorder to record audio/video. | |
int | streamrecorder_enable_source_buffer (streamrecorder_h recorder, streamrecorder_source_e type) |
Sets the video source as live buffer to be used for recording. | |
int | streamrecorder_get_state (streamrecorder_h recorder, streamrecorder_state_e *state) |
Gets the streamrecorder's current state. | |
Typedefs | |
typedef struct streamrecorder_s * | streamrecorder_h |
The Streamrecorder handle. |
Typedef Documentation
typedef struct streamrecorder_s* streamrecorder_h |
The Streamrecorder handle.
- Since :
- 3.0
Enumeration Type Documentation
Enumeration for Streamrecorder error type.
- Since :
- 3.0
- Enumerator:
Enumeration for the file container format.
- Since :
- 3.0
Enumeration for Streamrecorder states.
- Since :
- 3.0
- Enumerator:
Enumeration for the pixel format.
- Since :
- 3.0
- Enumerator:
STREAMRECORDER_VIDEO_SOURCE_FORMAT_INVALID Invalid pixel format
STREAMRECORDER_VIDEO_SOURCE_FORMAT_NV12 NV12 pixel format
STREAMRECORDER_VIDEO_SOURCE_FORMAT_NV21 NV21 pixel format
STREAMRECORDER_VIDEO_SOURCE_FORMAT_I420 I420 pixel format
STREAMRECORDER_VIDEO_SOURCE_FORMAT_NUM - Deprecated:
- Number of the video source format (Deprecated since 6.0, please don't use it)
Function Documentation
int streamrecorder_cancel | ( | streamrecorder_h | recorder | ) |
Cancels the recording.
The recording data is discarded and not written in the recording file.
- Since :
- 3.0
- Remarks:
- When you want to record audio or video file, you need to add privilege according to rules below additionally.
http://tizen.org/privilege/mediastorage is needed if input or output path are relevant to media storage.
http://tizen.org/privilege/externalstorage is needed if input or output path are relevant to external storage.
- Parameters:
-
[in] recorder The handle to the streamrecorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STREAMRECORDER_ERROR_NONE Successful STREAMRECORDER_ERROR_NOT_SUPPORTED Not supported STREAMRECORDER_ERROR_INVALID_PARAMETER Invalid parameter STREAMRECORDER_ERROR_INVALID_OPERATION Invalid operation STREAMRECORDER_ERROR_INVALID_STATE Invalid state STREAMRECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted
- Precondition:
- Create a stream recorder handle by calling streamrecorder_create().
- The streamrecorder state must be STREAMRECORDER_STATE_RECORDING set by streamrecorder_start() or STREAMRECORDER_STATE_PAUSED by streamrecorder_pause().
- Postcondition:
- The streamrecorder state will be STREAMRECORDER_STATE_PREPARED.
int streamrecorder_commit | ( | streamrecorder_h | recorder | ) |
Stops recording and saves the result.
- Since :
- 3.0
- Remarks:
- When you want to record audio or video file, you need to add privilege according to rules below additionally.
http://tizen.org/privilege/mediastorage is needed if input or output path are relevant to media storage.
http://tizen.org/privilege/externalstorage is needed if input or output path are relevant to external storage.
- Parameters:
-
[in] recorder The handle to the streamrecorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STREAMRECORDER_ERROR_NONE Successful STREAMRECORDER_ERROR_NOT_SUPPORTED Not supported STREAMRECORDER_ERROR_INVALID_PARAMETER Invalid parameter STREAMRECORDER_ERROR_INVALID_OPERATION Invalid operation STREAMRECORDER_ERROR_INVALID_STATE Invalid state STREAMRECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted
- Precondition:
- Create a stream recorder handle by calling streamrecorder_create().
- The streamrecorder state must be STREAMRECORDER_STATE_RECORDING set by streamrecorder_start() or STREAMRECORDER_STATE_PAUSED by streamrecorder_pause().
- Postcondition:
- The streamrecorder state will be STREAMRECORDER_STATE_PREPARED.
int streamrecorder_create | ( | streamrecorder_h * | recorder | ) |
Creates a streamrecorder handle to record a video or audio.
- Since :
- 3.0
- Remarks:
- You must release recorder using streamrecorder_destroy().
- Parameters:
-
[out] recorder A handle to the streamrecorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STREAMRECORDER_ERROR_NONE Successful STREAMRECORDER_ERROR_NOT_SUPPORTED Not supported STREAMRECORDER_ERROR_INVALID_PARAMETER Invalid parameter STREAMRECORDER_ERROR_OUT_OF_MEMORY Out of memory STREAMRECORDER_ERROR_INVALID_OPERATION Invalid operation
int streamrecorder_destroy | ( | streamrecorder_h | recorder | ) |
Destroys the streamrecorder handle.
- Since :
- 3.0
- Parameters:
-
[in] recorder The handle to the streamrecorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STREAMRECORDER_ERROR_NONE Successful STREAMRECORDER_ERROR_NOT_SUPPORTED Not supported STREAMRECORDER_ERROR_INVALID_PARAMETER Invalid parameter STREAMRECORDER_ERROR_INVALID_OPERATION Invalid operation STREAMRECORDER_ERROR_INVALID_STATE Invalid state
- Precondition:
- Create a stream recorder handle by calling streamrecorder_create().
- The recorder state should be STREAMRECORDER_STATE_CREATED.
- Postcondition:
- The recorder state will be STREAMRECORDER_STATE_NONE.
- See also:
- streamrecorder_create()
int streamrecorder_enable_source_buffer | ( | streamrecorder_h | recorder, |
streamrecorder_source_e | type | ||
) |
Sets the video source as live buffer to be used for recording.
- Since :
- 3.0
- Remarks:
- if you want to enable video or audio or both recording, call before streamrecorder_prepare()
- Parameters:
-
[in] recorder A handle to the streamrecorder [in] type The type of source input
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STREAMRECORDER_ERROR_NONE Successful STREAMRECORDER_ERROR_NOT_SUPPORTED Not supported STREAMRECORDER_ERROR_INVALID_PARAMETER Invalid parameter STREAMRECORDER_ERROR_INVALID_STATE Invalid state
- Precondition:
- Create a stream recorder handle by calling streamrecorder_create().
- The streamrecorder state must be only STREAMRECORDER_STATE_CREATED
- See also:
- streamrecorder_create()
int streamrecorder_get_state | ( | streamrecorder_h | recorder, |
streamrecorder_state_e * | state | ||
) |
Gets the streamrecorder's current state.
- Since :
- 3.0
- Parameters:
-
[in] recorder The handle to the streamrecorder [out] state The current state of the streamrecorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STREAMRECORDER_ERROR_NONE Successful STREAMRECORDER_ERROR_NOT_SUPPORTED Not supported STREAMRECORDER_ERROR_INVALID_PARAMETER Invalid parameter
- Precondition:
- Create a stream recorder handle by calling streamrecorder_create().
- See also:
- streamrecorder_create()
int streamrecorder_pause | ( | streamrecorder_h | recorder | ) |
Pauses the recording.
- Since :
- 3.0
- Remarks:
- Recording can be resumed with streamrecorder_start().
- Parameters:
-
[in] recorder The handle to the streamrecorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STREAMRECORDER_ERROR_NONE Successful STREAMRECORDER_ERROR_NOT_SUPPORTED Not supported STREAMRECORDER_ERROR_INVALID_PARAMETER Invalid parameter STREAMRECORDER_ERROR_INVALID_OPERATION Invalid operation STREAMRECORDER_ERROR_INVALID_STATE Invalid state
- Precondition:
- Create a stream recorder handle by calling streamrecorder_create().
- The streamrecorder state must be STREAMRECORDER_STATE_RECORDING.
- Postcondition:
- The streamrecorder state will be STREAMRECORDER_STATE_PAUSED.
int streamrecorder_prepare | ( | streamrecorder_h | recorder | ) |
Prepares the streamrecorder for recording.
- Since :
- 3.0
- Remarks:
- Before calling the function, it is required to properly set streamrecorder_enable_source_buffer(), audio encoder (streamrecorder_set_audio_encoder()), video encoder(streamrecorder_set_video_encoder()) and file format (streamrecorder_set_file_format()).
- Parameters:
-
[in] recorder The handle to the streamrecorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STREAMRECORDER_ERROR_NONE Successful STREAMRECORDER_ERROR_NOT_SUPPORTED Not supported STREAMRECORDER_ERROR_INVALID_PARAMETER Invalid parameter STREAMRECORDER_ERROR_INVALID_OPERATION Invalid operation STREAMRECORDER_ERROR_INVALID_STATE Invalid state
- Precondition:
- Create a stream recorder handle by calling streamrecorder_create().
-
The recorder state should be STREAMRECORDER_STATE_CREATED.
The streamrecorder_enable_source_buffer() should be invoked before this function.
- Postcondition:
- The streamrecorder state will be STREAMRECORDER_STATE_PREPARED
int streamrecorder_push_stream_buffer | ( | streamrecorder_h | recorder, |
media_packet_h | inbuf | ||
) |
Pushes buffer to StreamRecorder to record audio/video.
- Since :
- 3.0
- Remarks:
- When you want to record audio or video file, you need to add privilege according to rules below additionally.
http://tizen.org/privilege/mediastorage is needed if input or output path are relevant to media storage.
http://tizen.org/privilege/externalstorage is needed if input or output path are relevant to external storage.
- Parameters:
-
[in] recorder The handle to the streamrecorder [in] inbuf The media packet containing buffer and other associated values
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STREAMRECORDER_ERROR_NONE Successful STREAMRECORDER_ERROR_NOT_SUPPORTED Not supported STREAMRECORDER_ERROR_INVALID_PARAMETER Invalid parameter STREAMRECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted
- Precondition:
- Create a stream recorder handle by calling streamrecorder_create().
- See also:
- streamrecorder_create()
int streamrecorder_start | ( | streamrecorder_h | recorder | ) |
Starts the recording.
- Since :
- 3.0
- Remarks:
- If file path has been set to an existing file, this file is removed automatically and updated by new one.
When you want to record audio or video file, you need to add privilege according to rules below additionally.
http://tizen.org/privilege/mediastorage is needed if input or output path are relevant to media storage.
http://tizen.org/privilege/externalstorage is needed if input or output path are relevant to external storage. The filename should be set before this function is invoked.
- Parameters:
-
[in] recorder The handle to the streamrecorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STREAMRECORDER_ERROR_NONE Successful STREAMRECORDER_ERROR_NOT_SUPPORTED Not supported STREAMRECORDER_ERROR_INVALID_PARAMETER Invalid parameter STREAMRECORDER_ERROR_INVALID_OPERATION Invalid operation STREAMRECORDER_ERROR_INVALID_STATE Invalid state STREAMRECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted
- Precondition:
- Create a stream recorder handle by calling streamrecorder_create().
-
The streamrecorder state must be STREAMRECORDER_STATE_PREPARED by streamrecorder_prepare() or STREAMRECORDER_STATE_PAUSED by streamrecorder_pause().
The filename should be set by streamrecorder_set_filename().
- Postcondition:
- The recorder state will be STREAMRECORDER_STATE_RECORDING.
int streamrecorder_unprepare | ( | streamrecorder_h | recorder | ) |
Resets the streamrecorder.
- Since :
- 3.0
- Parameters:
-
[in] recorder The handle to the streamrecorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STREAMRECORDER_ERROR_NONE Successful STREAMRECORDER_ERROR_NOT_SUPPORTED Not supported STREAMRECORDER_ERROR_INVALID_PARAMETER Invalid parameter STREAMRECORDER_ERROR_INVALID_OPERATION Invalid operation STREAMRECORDER_ERROR_INVALID_STATE Invalid state
- Precondition:
- Create a stream recorder handle by calling streamrecorder_create().
- The streamrecorder state should be STREAMRECORDER_STATE_PREPARED set by streamrecorder_prepare(), streamrecorder_cancel() or streamrecorder_commit().
- Postcondition:
- The streamrecorder state will be STREAMRECORDER_STATE_CREATED.