Tizen Native API
5.0
|
Capture snapshots the current scene and save as a file. More...
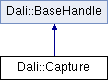
Public Types | |
enum | FinishState |
The enumerations used for checking capture success. More... | |
typedef Signal< void(Capture, Capture::FinishState) > | CaptureFinishedSignalType |
Typedef for finished signals sent by this class. | |
Public Member Functions | |
Capture () | |
Create an uninitialized Capture; this can be initialized with Actor::New(). | |
~Capture () | |
Dali::Actor is intended as a base class. | |
Capture (const Capture ©) | |
This copy constructor is required for (smart) pointer semantics. | |
Capture & | operator= (const Capture &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
void | Start (Actor source, const Vector2 &size, const std::string &path, const Vector4 &clearColor) |
Start capture and save the image as a file. | |
void | Start (Actor source, const Vector2 &size, const std::string &path) |
Start capture and save the image as a file. | |
CaptureFinishedSignalType & | FinishedSignal () |
Get finished signal. | |
Static Public Member Functions | |
static Capture | New () |
Create an initialized Capture. | |
static Capture | New (Dali::CameraActor cameraActor) |
Create an initialized Capture. | |
static Capture | DownCast (BaseHandle handle) |
Downcast an Object handle to Capture handle. |
Detailed Description
Capture snapshots the current scene and save as a file.
- Since:
- 4.0, DALi version 1.3.4
Applications should follow the example below to create capture :
Capture capture = Capture::New();
If required, you can also connect class member function to a signal :
capture.FinishedSignal().Connect(this, &CaptureSceneExample::OnCaptureFinished);
At the connected class member function, you can know whether capture finish state.
void CaptureSceneExample::OnCaptureFinished( Capture capture, Capture::FinishState state ) { if ( state == Capture::FinishState::SUCCEEDED ) { // Do something } else { // Do something } }
Member Typedef Documentation
typedef Signal< void ( Capture, Capture::FinishState ) > Dali::Capture::CaptureFinishedSignalType |
Typedef for finished signals sent by this class.
- Since:
- 4.0, DALi version 1.3.4
Member Enumeration Documentation
Constructor & Destructor Documentation
Create an uninitialized Capture; this can be initialized with Actor::New().
- Since:
- 4.0, DALi version 1.3.4
Calling member functions with an uninitialized Dali::Object is not allowed.
Dali::Actor is intended as a base class.
- Since:
- 4.0, DALi version 1.3.4
This is non-virtual since derived Handle types must not contain data or virtual methods.
Dali::Capture::Capture | ( | const Capture & | copy | ) |
This copy constructor is required for (smart) pointer semantics.
- Since:
- 4.0, DALi version 1.3.4
- Parameters:
-
[in] copy A reference to the copied handle.
Member Function Documentation
static Capture Dali::Capture::DownCast | ( | BaseHandle | handle | ) | [static] |
Get finished signal.
- Since:
- 4.0, DALi version 1.3.4
- Returns:
- finished signal instance.
static Capture Dali::Capture::New | ( | ) | [static] |
Create an initialized Capture.
- Since:
- 4.0, DALi version 1.3.4
- Returns:
- A handle to a newly allocated Dali resource.
- Note:
- Projection mode of default cameraActor is Dali::Camera::PERSPECTIVE_PROJECTION
static Capture Dali::Capture::New | ( | Dali::CameraActor | cameraActor | ) | [static] |
Create an initialized Capture.
- Since:
- 4.0, DALi version 1.3.4
- Parameters:
-
[in] cameraActor An initialized CameraActor.
- Returns:
- A handle to a newly allocated Dali resource.
This assignment operator is required for (smart) pointer semantics.
- Since:
- 4.0, DALi version 1.3.4
- Parameters:
-
[in] rhs A reference to the copied handle.
- Returns:
- A reference to this.
void Dali::Capture::Start | ( | Actor | source, |
const Vector2 & | size, | ||
const std::string & | path, | ||
const Vector4 & | clearColor | ||
) |
Start capture and save the image as a file.
- Since:
- 4.0, DALi version 1.3.4
- Parameters:
-
[in] source source actor to be used for capture. [in] size captured size. [in] path image file path to be saved as a file. [in] clearColor background color of captured scene
void Dali::Capture::Start | ( | Actor | source, |
const Vector2 & | size, | ||
const std::string & | path | ||
) |
Start capture and save the image as a file.
- Since:
- 4.0, DALi version 1.3.4
- Parameters:
-
[in] source source actor to be used for capture. [in] size captured size. [in] path image file path to be saved as a file.
- Note:
- Clear color is transparent.