Tizen Native API
3.0
|
Touch events are a collection of points at a specific moment in time. More...
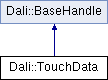
Public Member Functions | |
TouchData () | |
An uninitialized TouchData instance. | |
TouchData (const TouchData &other) | |
Copy constructor. | |
~TouchData () | |
Destructor. | |
TouchData & | operator= (const TouchData &other) |
Assignment Operator. | |
unsigned long | GetTime () const |
Returns the time (in ms) that the touch event occurred. | |
std::size_t | GetPointCount () const |
Returns the total number of points in this TouchData. | |
int32_t | GetDeviceId (std::size_t point) const |
Returns the ID of the device used for the Point specified. | |
PointState::Type | GetState (std::size_t point) const |
Retrieves the State of the point specified. | |
Actor | GetHitActor (std::size_t point) const |
Retrieves the actor that was underneath the point specified. | |
const Vector2 & | GetLocalPosition (std::size_t point) const |
Retrieves the co-ordinates relative to the top-left of the hit-actor at the point specified. | |
const Vector2 & | GetScreenPosition (std::size_t point) const |
Retrieves the co-ordinates relative to the top-left of the screen of the point specified. | |
float | GetRadius (std::size_t point) const |
Retrieves the radius of the press point. | |
const Vector2 & | GetEllipseRadius (std::size_t point) const |
Retrieves BOTH the horizontal and the vertical radii of the press point. | |
float | GetPressure (std::size_t point) const |
Retrieves the touch pressure. | |
Degree | GetAngle (std::size_t point) const |
Retrieves the angle of the press point relative to the Y-Axis. |
Detailed Description
Touch events are a collection of points at a specific moment in time.
When a multi-touch event occurs, each point represents the points that are currently being touched or the points where a touch has stopped.
The first point is the primary point that's used for hit-testing.
- Since:
- 3.0, DALi version 1.1.37
- Note:
- Should not use this in a TouchData container as it is just a handle and the internal object can change.
Constructor & Destructor Documentation
Dali::TouchData::TouchData | ( | const TouchData & | other | ) |
Destructor.
- Since:
- 3.0, DALi version 1.1.37
Member Function Documentation
Degree Dali::TouchData::GetAngle | ( | std::size_t | point | ) | const |
Retrieves the angle of the press point relative to the Y-Axis.
- Since:
- 3.0, DALi version 1.1.39
- Parameters:
-
[in] point The point required
- Returns:
- The angle of the press point
- Note:
- If point is greater than GetPointCount() then this method will return Degree().
int32_t Dali::TouchData::GetDeviceId | ( | std::size_t | point | ) | const |
Returns the ID of the device used for the Point specified.
Each point has a unique device ID which specifies the device used for that point. This is returned by this method.
- Since:
- 3.0, DALi version 1.1.37
- Parameters:
-
[in] point The point required
- Returns:
- The Device ID of this point
- Note:
- If point is greater than GetPointCount() then this method will return -1.
const Vector2& Dali::TouchData::GetEllipseRadius | ( | std::size_t | point | ) | const |
Retrieves BOTH the horizontal and the vertical radii of the press point.
- Since:
- 3.0, DALi version 1.1.39
- Parameters:
-
[in] point The point required
- Returns:
- The horizontal and vertical radii of the press point
- Note:
- If point is greater than GetPointCount() then this method will return Vector2::ZERO.
Actor Dali::TouchData::GetHitActor | ( | std::size_t | point | ) | const |
Retrieves the actor that was underneath the point specified.
- Since:
- 3.0, DALi version 1.1.37
- Parameters:
-
[in] point The point required
- Returns:
- The actor that was underneath the point specified
- Note:
- If point is greater than GetPointCount() then this method will return an empty handle.
const Vector2& Dali::TouchData::GetLocalPosition | ( | std::size_t | point | ) | const |
Retrieves the co-ordinates relative to the top-left of the hit-actor at the point specified.
- Since:
- 3.0, DALi version 1.1.37
- Parameters:
-
[in] point The point required
- Returns:
- The co-ordinates relative to the top-left of the hit-actor of the point specified
- Note:
- The top-left of an actor is (0.0, 0.0, 0.5).
- If you require the local coordinates of another actor (e.g the parent of the hit actor), then you should use Actor::ScreenToLocal().
- If point is greater than GetPointCount() then this method will return Vector2::ZERO.
std::size_t Dali::TouchData::GetPointCount | ( | ) | const |
Returns the total number of points in this TouchData.
- Since:
- 3.0, DALi version 1.1.37
- Returns:
- Total number of Points
float Dali::TouchData::GetPressure | ( | std::size_t | point | ) | const |
Retrieves the touch pressure.
The pressure range starts at 0.0f. Normal pressure is defined as 1.0f. A value between 0.0f and 1.0f means light pressure has been applied. A value greater than 1.0f means more pressure than normal has been applied.
- Since:
- 3.0, DALi version 1.1.39
- Parameters:
-
[in] point The point required
- Returns:
- The touch pressure
- Note:
- If point is greater than GetPointCount() then this method will return 1.0f.
float Dali::TouchData::GetRadius | ( | std::size_t | point | ) | const |
Retrieves the radius of the press point.
This is the average of both the horizontal and vertical radii of the press point.
- Since:
- 3.0, DALi version 1.1.39
- Parameters:
-
[in] point The point required
- Returns:
- The radius of the press point
- Note:
- If point is greater than GetPointCount() then this method will return 0.0f.
const Vector2& Dali::TouchData::GetScreenPosition | ( | std::size_t | point | ) | const |
Retrieves the co-ordinates relative to the top-left of the screen of the point specified.
- Since:
- 3.0, DALi version 1.1.37
- Parameters:
-
[in] point The point required
- Returns:
- The co-ordinates relative to the top-left of the screen of the point specified
- Note:
- If point is greater than GetPointCount() then this method will return Vector2::ZERO.
PointState::Type Dali::TouchData::GetState | ( | std::size_t | point | ) | const |
Retrieves the State of the point specified.
- Since:
- 3.0, DALi version 1.1.37
- Parameters:
-
[in] point The point required
- Returns:
- The state of the point specified
- Note:
- If point is greater than GetPointCount() then this method will return PointState::FINISHED.
- See also:
- State
unsigned long Dali::TouchData::GetTime | ( | ) | const |
Returns the time (in ms) that the touch event occurred.
- Since:
- 3.0, DALi version 1.1.37
- Returns:
- The time (in ms) that the touch event occurred