Navigation
The navigation component is used inside the header to navigate back and forth according to the navigational history array, which is created by the application. By clicking a horizontally-listed element on the navigation, you can move to the target page.
Note |
---|
This component is supported since Tizen 2.3. |
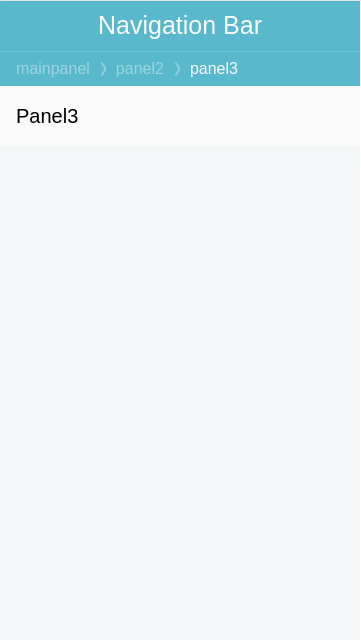
Table of Contents
Default Selector
Make the navigation component with the class="ui-navigation"
or data-role="navigation"
attribute. For semantic understanding, use the nav
element.
<div class="ui-page"> <div class="ui-header" data-position="fixed"> <h1>title</h1> <nav class="ui-navigation" id="navigation"></nav> </div> <div class="ui-content"></div> </div>
HTML Examples
Note |
---|
To get more efficient experience, aWe recommend to use Navigation component with PanelChanger. |
HTML example with PanelChanger
<div id="panelPage" class="ui-page"> <div class="ui-header" data-position="fixed"> <h1>Navigation</h1> <nav id="navigation" class="ui-navigation"> </nav> </div> <div class="ui-content"> <div id="panelChanger" class="ui-panel-changer"> <div id="mainpanel" class="ui-panel"> <a href="#panel2">Go Panel 2</a> </div> </div> </div> <script src="./panel.js"></script> </div> <!--panel.html--> <div id="panel2" class="ui-panel"> <div class="ui-content"> <ul class="ui-listview"> <li class="ui-li-anchor"> <a href="#panel3">Go Panel 3</a> </li> </ul> </div> </div> <div id="panel3" class="ui-panel"> <div class="ui-content"> <ul class="ui-listview"> <li class="ui-li-anchor"> <a>Panel3</a> </li> </ul> </div> </div>
JS example with PanelChanger
/* panel.js */ (function() { var page = document.querySelector("#panelPage"), panelChanger = page.querySelector("#panelChanger"), panelChangerComponent, navigation = page.querySelector("#navigation"), navigationComponent, navigated = false; page.addEventListener("pagebeforeshow", function() { var activePanel = page.querySelector(".ui-panel-active"); panelChangerComponent = tau.widget.PanelChanger(panelChanger); navigationComponent = tau.widget.Navigation(navigation); navigationComponent.push(activePanel.id); }); panelChanger.addEventListener("panelchange", function(event) { var toPanel = event.detail.toPanel, direction = event.detail.direction, id = toPanel.id; if (id) { if (direction === "forward") { navigationComponent.push(id); } else { if (navigated === false) { navigationComponent.pop(); } else { navigated = false; } } } else { console.warn("You should insert id value in the each panels") } }); navigation.addEventListener("navigate", function(event) { var id = event.detail.id; navigated = true; panelChangerComponent.changePanel("#" + id, "slide-reverse", "back"); }); })();
Methods
Summary
Method | Description |
---|---|
create() |
Initiates the making of the navigation. |
push(String targetId) |
Add navigation item only one at a time. |
pop() |
Pop last child of navigation. |
create :DEPRECATED since 2.4
-
Initiates the making of the navigation.
create()
push
-
Add navigation item only one at a time.
push(String targetId)
Parameters:
Parameter Type Required / optional Description targetId String required element id to be added Return value:
No return valueCode example:
HTML code:
<div class="ui-header" data-position="fixed"> <h1>Navigation</h1> <nav id="navigation" class="ui-navigation"> </nav> </div> <div class="ui-content"> <div id="panelChanger" class="ui-panel-changer"> <div id="mainpanel" class="ui-panel" style="background-color:#ffff4f"> <a href="./panel.html#panel2">Go Panel 2</a> </div> </div> </div>
JS code:
var navigation = page.querySelector("#navigation"), activePanel = page.querySelector(".ui-panel-active"), navigationComponent = tau.widget.Navigation(navigation); navigationComponent.push(activePanel.id);
pop
-
Pop last child of navigation.
pop()
Return value:
No return valueCode example:
HTML code:
<div class="ui-header" data-position="fixed"> <h1>Navigation</h1> <nav id="navigation" class="ui-navigation"> </nav> </div>
JS code:
var navigation = page.querySelector("#navigation"), navigationComponent = tau.widget.Navigation(navigation); navigationComponent.pop();