Tizen Native API
7.0
|
The Voice control elementary API provides functions to control widget by voice commands.
Required Header
#include <voice_control_elm.h>
Overview
The voice control elementary API is provided for controlling widgets by voice commands. The voice UI control provided by voice control elementary is done the following way:
- Each widget supported and currently visible in the application is given a text hint.
- Widget hints are shown on the User's wish.
- User speaks the hint name of the widget he/she wants to trigger and the action he/she wants to perform on that widget.
- The voice command is interpreted and the requested action on the widget is performed.
To use of voice control elementary, use the following steps:
1. Initialize voice control elementary
2. Register callback functions for notifications
3. Create a handle after creating an evas object or elementary object item
4. Set command and hint
5. Set hint direction if you want to change hint position
6. Run action mapped widget which is spoken by user
7. Destroy handle
8. Uninitialize voice control elementary
When window is updated by user's operation with touch, key or etc, it is needed to set command and hint creating evas object or elementary object item.
And the callback function of action related to widget is called internally, when user speaks a particular widget's hint.
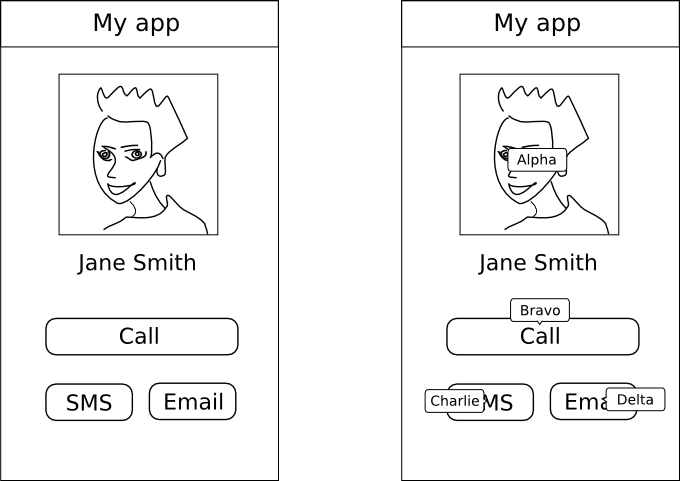
Here is an example code for supporting voice control elm module :
#include <app.h> #include <voice_control_elm.h> void app_control(app_control_h app_control, void *user_data) { vc_elm_initialize(); vc_elm_set_current_language_changed_cb(_vc_elm_language_changed_cb, NULL); elm_main(0, (char **)NULL); } void app_terminate(void *user_data) { vc_elm_deinitialize(); } int main(int argc, char *argv[]) { struct appdata ad; ui_app_lifecycle_callback_s event_callback = {0,}; event_callback.create = app_create; event_callback.terminate = app_terminate; event_callback.pause = NULL; event_callback.resume = NULL; event_callback.app_control = NULL; memset(&ad, 0x0, sizeof(struct appdata)); return ui_app_main(argc, argv, &event_callback, &ad); }
If you want to create some widgets with voice control, you can refer below function.
int elm_main(int argc, char **argv) { Evas_Object* win; Evas_Object* btn; vc_elm_h vc_elm_buttons_h; win = elm_win_add(NULL, "example", ELM_WIN_BASIC); elm_win_title_set(win, "example"); elm_win_autodel_set(win, EINA_TRUE); evas_object_resize(win, 720, 1280); evas_object_show(win); btn = elm_button_add(win); elm_object_text_set(btn, "Button"); evas_object_move(btn, 50, 50); evas_object_resize(btn, 200, 50); vc_elm_create_object(btn, &vc_elm_buttons_h); vc_elm_set_command(vc_elm_buttons_h, "Button"); vc_elm_set_command_hint(vc_elm_buttons_h, "Button"); evas_object_show(btn); return 0; }
If you want to support several language for voice control elm module, you need to set language changed callback function.
void _vc_elm_language_changed_cb(void) { // You need to update codes in order to change text of command & hint according to changed language. }
Related Features
This API is related with the following features:
- http://tizen.org/feature/microphone
- http://tizen.org/feature/speech.control
It is recommended to design feature related codes in your application for reliability.
You can check if a device supports the related features for this API by using System Information, thereby controlling the procedure of your application.
To ensure your application is only running on the device with specific features, please define the features in your manifest file using the manifest editor in the SDK.
More details on featuring your application can be found from Feature Element.
Functions | |
int | vc_elm_initialize (void) |
Initializes voice control elementary module. | |
int | vc_elm_deinitialize (void) |
Deinitializes voice control elementary module. | |
int | vc_elm_foreach_supported_languages (vc_elm_supported_language_cb callback, void *user_data) |
Retrieves all supported languages using callback function. | |
int | vc_elm_get_current_language (char **language) |
Gets current language. | |
int | vc_elm_foreach_supported_widgets (vc_elm_widget_cb callback, void *user_data) |
Retrieves all supported widget using callback function. | |
int | vc_elm_foreach_supported_actions (const char *widget, vc_elm_action_cb callback, void *user_data) |
Retrieves all supported actions of widget using callback function. | |
int | vc_elm_get_action_command (const char *action, char **command) |
Gets action command of action. | |
int | vc_elm_create_object (Evas_Object *object, vc_elm_h *vc_elm) |
Creates vc elm handle for Evas object. | |
int | vc_elm_create_item (Elm_Object_Item *item, vc_elm_h *vc_elm) |
Creates vc elm handle for elm object item. | |
int | vc_elm_destroy (vc_elm_h vc_elm) |
Destroys the handle. | |
int | vc_elm_set_command (vc_elm_h vc_elm, const char *command) |
Sets command to the handle. | |
int | vc_elm_unset_command (vc_elm_h vc_elm) |
Unsets command from the handle. | |
int | vc_elm_set_command_hint (vc_elm_h vc_elm, const char *hint) |
Sets command hint for the handle. | |
int | vc_elm_unset_command_hint (vc_elm_h vc_elm) |
Unsets command hint for the handle. | |
int | vc_elm_set_command_hint_direction (vc_elm_h vc_elm, vc_elm_direction_e direction) |
Sets the direction of hint to the handle. | |
int | vc_elm_get_command_hint_direction (vc_elm_h vc_elm, vc_elm_direction_e *direction) |
Unsets the direction of hint from the handle. | |
int | vc_elm_set_command_hint_offset (vc_elm_h vc_elm, int pos_x, int pos_y) |
Sets command hint's x,y position to the handle. | |
int | vc_elm_get_command_hint_offset (vc_elm_h vc_elm, int *pos_x, int *pos_y) |
Gets command hint's x,y position from the handle. | |
int | vc_elm_set_current_language_changed_cb (vc_elm_current_language_changed_cb callback, void *user_data) |
Registers a callback function to be called when current language is changed. | |
int | vc_elm_unset_current_language_changed_cb (void) |
Unregisters the callback function. | |
Typedefs | |
typedef struct vc_elm_s * | vc_elm_h |
The voice control elementary for object or item object handle. | |
typedef bool(* | vc_elm_supported_language_cb )(const char *language, void *user_data) |
Called to retrieve supported language. | |
typedef void(* | vc_elm_current_language_changed_cb )(const char *previous, const char *current, void *user_data) |
Called when default language is changed. | |
typedef bool(* | vc_elm_widget_cb )(const char *widget, void *user_data) |
Called to retrieve supported widget. | |
typedef bool(* | vc_elm_action_cb )(const char *action, void *user_data) |
Called to retrieve supported action. |
Typedef Documentation
typedef bool(* vc_elm_action_cb)(const char *action, void *user_data) |
Called to retrieve supported action.
- Since :
- 2.4
- Parameters:
-
[in] action Action name [in] user_data The user data passed from the callback registration function
- Returns:
true
to continue with the next iteration of the loop,false
to break out of the loop
- Precondition:
- The function will invoke this callback.
- See also:
- vc_elm_foreach_supported_actions()
typedef void(* vc_elm_current_language_changed_cb)(const char *previous, const char *current, void *user_data) |
Called when default language is changed.
- Since :
- 2.4
- Parameters:
-
[in] previous Previous language [in] current Current language [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers this callback to detect changing language.
typedef struct vc_elm_s* vc_elm_h |
The voice control elementary for object or item object handle.
- Since :
- 2.4
typedef bool(* vc_elm_supported_language_cb)(const char *language, void *user_data) |
Called to retrieve supported language.
- Since :
- 2.4
- Parameters:
-
[in] language A language is specified as an ISO 3166 alpha-2 two letter country-code followed by ISO 639-1 for the two-letter language code For example, "ko_KR" for Korean, "en_US" for American English [in] user_data The user data passed from the foreach function
- Returns:
true
to continue with the next iteration of the loop,false
to break out of the loop
- Precondition:
- The function will invoke this callback.
- See also:
- vc_elm_foreach_supported_languages()
typedef bool(* vc_elm_widget_cb)(const char *widget, void *user_data) |
Called to retrieve supported widget.
- Since :
- 2.4
- Parameters:
-
[in] widget Widget name [in] user_data The user data passed from the callback registration function
- Returns:
true
to continue with the next iteration of the loop,false
to break out of the loop
- Precondition:
- The function will invoke this callback.
- See also:
- vc_elm_foreach_supported_widgets()
Enumeration Type Documentation
enum vc_elm_direction_e |
Enumeration for directions of the widget hints.
- Since :
- 2.4
- See also:
- vc_elm_set_command_hint_direction() Example: various hints orientations.
- Enumerator:
enum vc_elm_error_e |
Enumeration for status of voice control elementary after API call.
- Since :
- 2.4
- Enumerator:
Function Documentation
int vc_elm_create_item | ( | Elm_Object_Item * | item, |
vc_elm_h * | vc_elm | ||
) |
Creates vc elm handle for elm object item.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If the function succeeds, The vc elm handle must be released with vc_elm_destroy().
- Parameters:
-
[in] item The elm Object item included in handle [out] vc_elm Handle containing pointer to widget
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_OUT_OF_MEMORY Out of memory VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- vc_elm_initialize() should be successful.
- See also:
- vc_elm_destroy()
int vc_elm_create_object | ( | Evas_Object * | object, |
vc_elm_h * | vc_elm | ||
) |
Creates vc elm handle for Evas object.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If the function succeeds, The vc elm handle must be released with vc_elm_destroy().
- Parameters:
-
[in] object Evas object included in handle [out] vc_elm The voice control elementary handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_OUT_OF_MEMORY Out of memory VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_OPERATION_REJECTED Operation rejected when widget is not supported VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- vc_elm_initialize() should be successful.
- See also:
- vc_elm_destroy()
int vc_elm_deinitialize | ( | void | ) |
Deinitializes voice control elementary module.
- Since :
- 2.4
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_OUT_OF_MEMORY Out of memory VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- vc_elm_initialize() should be successful.
- See also:
- vc_elm_initialize()
int vc_elm_destroy | ( | vc_elm_h | vc_elm | ) |
Destroys the handle.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] vc_elm Handle containing pointer to widget
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The vc elm handle should be valid with vc_elm_create_object() or vc_elm_create_item().
int vc_elm_foreach_supported_actions | ( | const char * | widget, |
vc_elm_action_cb | callback, | ||
void * | user_data | ||
) |
Retrieves all supported actions of widget using callback function.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] widget Widget name [in] callback Callback function to invoke [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- vc_elm_initialize() should be successful and widget name can get from vc_elm_foreach_supported_widgets().
- Postcondition:
- This function invokes vc_elm_action_cb() repeatedly for getting actions.
int vc_elm_foreach_supported_languages | ( | vc_elm_supported_language_cb | callback, |
void * | user_data | ||
) |
Retrieves all supported languages using callback function.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] callback Callback function to invoke [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- vc_elm_initialize() should be successful.
- Postcondition:
- This function invokes vc_supported_language_cb() repeatedly for getting languages.
int vc_elm_foreach_supported_widgets | ( | vc_elm_widget_cb | callback, |
void * | user_data | ||
) |
Retrieves all supported widget using callback function.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] callback Callback function to invoke [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- vc_elm_initialize() should be successful.
- Postcondition:
- This function invokes vc_elm_widget_cb() repeatedly for getting widgets.
int vc_elm_get_action_command | ( | const char * | action, |
char ** | command | ||
) |
Gets action command of action.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If the function succeeds, command must be released with free() by you when you no longer need it. If command is NULL, the action is default action. The default action works by only command of widget.
- Parameters:
-
[in] action Action name [out] command Command for action
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OUT_OF_MEMORY Out of memory VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- vc_elm_initialize() should be successful.
int vc_elm_get_command_hint_direction | ( | vc_elm_h | vc_elm, |
vc_elm_direction_e * | direction | ||
) |
Unsets the direction of hint from the handle.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] vc_elm Handle containing pointer to widget [out] direction Direction of hint defined by vc_elm_direction_e
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The vc_elm handle should be valid with vc_elm_create_object() or vc_elm_create_item().
- See also:
- vc_elm_set_command_hint_direction()
int vc_elm_get_command_hint_offset | ( | vc_elm_h | vc_elm, |
int * | pos_x, | ||
int * | pos_y | ||
) |
Gets command hint's x,y position from the handle.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] vc_elm Handle containing pointer to widget [out] pos_x The x position of hint [out] pos_y The y position of hint
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The vc_elm handle should be valid with vc_elm_create_object() or vc_elm_create_item().
- See also:
- vc_elm_set_command_hint_offset()
int vc_elm_get_current_language | ( | char ** | language | ) |
Gets current language.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If the function succeeds, language must be released with free() by you when you no longer need it.
- Parameters:
-
[out] language A language is specified as an ISO 3166 alpha-2 two letter country-code followed by ISO 639-1 for the two-letter language code For example, "ko_KR" for Korean, "en_US" for American English
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OUT_OF_MEMORY Out of memory VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- vc_elm_initialize() should be successful.
int vc_elm_initialize | ( | void | ) |
Initializes voice control elementary module.
- Since :
- 2.4
- Remarks:
- If the function succeeds, the voice control elementary must be released with vc_elm_deinitialize().
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_OUT_OF_MEMORY Out of memory VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_NOT_SUPPORTED Not supported
- See also:
- vc_elm_deinitialize()
int vc_elm_set_command | ( | vc_elm_h | vc_elm, |
const char * | command | ||
) |
Sets command to the handle.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] vc_elm Handle containing pointer to widget [in] command Command's text
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful. VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The vc_elm handle should be valid with vc_elm_create_object() or vc_elm_create_item().
- See also:
- vc_elm_unset_command()
int vc_elm_set_command_hint | ( | vc_elm_h | vc_elm, |
const char * | hint | ||
) |
Sets command hint for the handle.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] vc_elm Handle containing pointer to widget [in] hint Hint's text
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The vc_elm handle should be valid with vc_elm_create_object() or vc_elm_create_item().
- See also:
- vc_elm_unset_command_hint()
int vc_elm_set_command_hint_direction | ( | vc_elm_h | vc_elm, |
vc_elm_direction_e | direction | ||
) |
Sets the direction of hint to the handle.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If direction is not set, default direction is pre-configured will be used.
- Parameters:
-
[in] vc_elm Handle containing pointer to widget [in] direction Direction of hint defined by vc_elm_direction_e
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The vc_elm handle should be valid with vc_elm_create_object() or vc_elm_create_item().
int vc_elm_set_command_hint_offset | ( | vc_elm_h | vc_elm, |
int | pos_x, | ||
int | pos_y | ||
) |
Sets command hint's x,y position to the handle.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] vc_elm Handle containing pointer to widget [in] pos_x The x position of hint [in] pos_y The y position of hint
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The vc_elm handle should be valid with vc_elm_create_object() or vc_elm_create_item().
int vc_elm_set_current_language_changed_cb | ( | vc_elm_current_language_changed_cb | callback, |
void * | user_data | ||
) |
Registers a callback function to be called when current language is changed.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] callback Callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- vc_elm_initialize() should be successful.
int vc_elm_unset_command | ( | vc_elm_h | vc_elm | ) |
Unsets command from the handle.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] vc_elm Handle to include pointer to widget
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The vc_elm handle should be valid with vc_elm_create_object() or vc_elm_create_item().
- See also:
- vc_elm_set_command()
int vc_elm_unset_command_hint | ( | vc_elm_h | vc_elm | ) |
Unsets command hint for the handle.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] vc_elm Handle containing pointer to widget
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_PARAMETER Invalid parameter VC_ELM_ERROR_OPERATION_FAILED Operation failed VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The vc_elm handle should be valid with vc_elm_create_object() or vc_elm_create_item().
- See also:
- vc_elm_set_command_hint()
int vc_elm_unset_current_language_changed_cb | ( | void | ) |
Unregisters the callback function.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ELM_ERROR_NONE Successful VC_ELM_ERROR_INVALID_STATE Invalid state VC_ELM_ERROR_PERMISSION_DENIED Permission denied VC_ELM_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- vc_elm_initialize() should be successful.